Manage Room Mailbox permissions using PowerShell
In this article you will learn how to manage Room Mailbox permissions using PowerShell commands. You will learn how to assign permissions on Room Mailbox and Room Mailbox’s calendar.
Table of Contents
Manage Room Mailbox permissions using PowerShell
With PowerShell, you can swiftly configure and control access permissions for room mailboxes, ensuring seamless scheduling and resource allocation within your workspace. From granting booking rights to specific users to customizing delegate access, PowerShell offers a robust solution for optimizing room mailbox management, empowering administrators to efficiently oversee resource utilization while maintaining security and privacy standards.
Assign permissions on Room Mailbox using PowerShell commands
Connect to Exchange Online PowerShell
To be able to run below PowerShell commands, you need to connect to Exchange Online PowerShell module. Open Windows PowerShell as administrator and run below commands one by one:
Set-ExecutionPolicy -ExecutionPolicy RemoteSigned -Scope CurrentUser
Install-Module -Name ExchangeOnlineManagement
Import-Module ExchangeOnlineManagement
Connect-ExchangeOnline
Assign Full Access permission on a Room Mailbox using PowerShell
In this scenario we will assign Full Access permission to a user on Room Mailbox.
Add-MailboxPermission -Identity "Meeting-Room1" -User "ConceptsUser" -AccessRights FullAccess -InheritanceType All
The above command will assign Full Access permission to user “ConceptsUser” on Room Mailbox “Meeting-Room1”.
Assign Full Access permission on a Room Mailbox with Auto Mapping enabled
Full Access permission offers a feature Auto Mapping that automatically adds a mailbox to a user’s Outlook profile when Full Access permissions are granted to that user. This means that when a user is given Full Access permission to another mailbox (user mailbox/shared mailbox/room mailbox) along with Auto Mapping, Outlook will automatically display the room mailbox in their mailbox list without needing to manually add it.
To assign Full Access permission on a room mailbox along with Auto Mapping feature enabled, use below PowerShell command:
Add-MailboxPermission -Identity "Meeting-Room2" -User "ConceptsUser" -AccessRights FullAccess -InheritanceType All -AutoMapping $true
Bulk assign Full Access permission on Room Mailboxes using CSV and PowerShell
In this example, we will use CSV and PowerShell to assign Full Access permission to multiple users on Room Mailboxes.
Create a CSV using below columns:
UserName: This column will include the email address of each user who needs Full Access permission on the Room Mailbox.
RoomMailboxName: This column will include the display name or email address of each room mailbox.
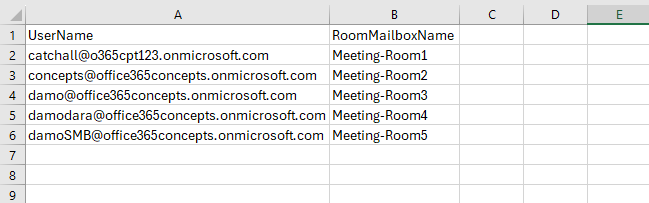
Replace “C:\csv files\RoomMailboxPermissions.csv” with the actual path to your CSV file and run below PowerShell script:
# Define the path to the CSV file
$csvPath = "C:\csv files\RoomMailboxPermissions.csv"
# Import the CSV file
$permissionList = Import-Csv -Path $csvPath
# Loop through each entry in the CSV file
foreach ($entry in $permissionList) {
$userName = $entry.UserName
$roomMailboxName = $entry.RoomMailboxName
# Assign Full Access permission to the user on the room mailbox
Add-MailboxPermission -Identity $roomMailboxName -User $userName -AccessRights FullAccess -InheritanceType All
Write-Host "Full Access permission granted to $userName on $roomMailboxName"
}
Bulk assign Full Access permission on Room Mailboxes using Custom Attribute
In this example, we will assign Full Access permission to a user on multiple Room Mailboxes whose CustomAttribute1 is set to “Test”.
To achieve this, we will first filter the Room Mailboxes using RecipientTypeDetails parameter, then we will fetch the Room Mailboxes for whom CustomAttribute1 is set to “Test”, and finally, we will use Add-MailboxPermission command to assign Full Access permission on those mailboxes to a user.
Replace “ConceptsUser” with the actual display name of the user who needs permission on the Room Mailbox, and run below PowerShell command:
# Define the username to grant full access permission
$userName = "ConceptsUser"
# Get room mailboxes with CustomAttribute1 set to "Test"
$roomMailboxes = Get-Mailbox -Filter {RecipientTypeDetails -eq "RoomMailbox" -and CustomAttribute1 -eq "Test"}
# Loop through each room mailbox and assign full access permission to the user
foreach ($roomMailbox in $roomMailboxes) {
# Assign Full Access permission to the user on the room mailbox
Add-MailboxPermission -Identity $roomMailbox.Identity -User $userName -AccessRights FullAccess -InheritanceType All -AutoMapping $false
Write-Host "Full Access permission granted to $userName on $($roomMailbox.Name)"
}
Bulk assign Full Access Permission on all Room Mailboxes to a user
In this example, we will assign Full Access permission to a user on all Room Mailboxes. To achieve this we will first filter the Room Mailboxes using RecipientTypeDetails parameter and then we will use Add-MailboxPermission command to assign Full Access permission on those room mailboxes to a user.
Replace “ConceptsUser” with the actual display name or email address of the user who needs Full Access permission on room mailbox and run below PowerShell script:
# Define the username to grant full access permission
$userName = "ConceptsUser"
# Get room mailboxes with CustomAttribute1 set to "Test"
$roomMailboxes = Get-Mailbox -Filter {RecipientTypeDetails -eq "RoomMailbox" }
# Loop through each room mailbox and assign full access permission to the user
foreach ($roomMailbox in $roomMailboxes) {
# Assign Full Access permission to the user on the room mailbox
Add-MailboxPermission -Identity $roomMailbox.Identity -User $userName -AccessRights FullAccess -AutoMapping $false -InheritanceType All
Write-Host "Full Access permission granted to $userName on $($roomMailbox.Name)"
}
Assign Send As permission on a Room Mailbox
In this example we will assign Send As permission to a user on a Room Mailbox.
Add-RecipientPermission -Identity "Meeting-Room1" -Trustee "ConceptsUser" -AccessRights SendAs
Assign Send As permission to each member of a group
In this example, we will assign Send As permission on a Room Mailbox to each member of a security group.
To achieve this we will fetch the members of the group, and then will loop through each member to assign the “Send As” permission on the specified room mailbox using the Add-RecipientPermission cmdlet.
Replace “Meeting-Room1” with the actual name of the Room Mailbox and “SecurityGroup” with the name of your security group, and run below PowerShell command:
# Specify the room mailbox and distribution group
$roomMailbox = "Meeting-Room1"
$distributionGroup = "SecurityGroup"
# Get members of the distribution group
$groupMembers = Get-DistributionGroupMember -Identity $distributionGroup
# Loop through each member and assign "Send As" permission on the room mailbox
foreach ($member in $groupMembers) {
Add-RecipientPermission -Identity $roomMailbox -Trustee $member.PrimarySmtpAddress -AccessRights SendAs -Confirm:$false
Write-Host "Send As permission assigned to $($member.PrimarySmtpAddress) on $roomMailbox"
}
Assign Send on Behalf permission on a Room Mailbox
In this example, we will assign Send on Behalf permission to a user on a Room Mailbox.
Set-Mailbox -Identity "Meeting-Room1" -GrantSendOnBehalfTo "ConceptsUser" -Confirm:$false
Assign permissions on Room Mailbox calendar using PowerShell
Assign Author permission on Room Mailbox Calendar
In this example we will assign Author permission to a user on Room Mailbox calendar. You can assign permissions on a mailbox calendar using Add-MailboxFolderPermission PowerShell command.
Add-MailboxFolderPermission -Identity "Meeting-Room1:\Calendar" -User "ConceptsUser" -AccessRights Author -Confirm:$false
Assign Availability Only permission on Room Mailbox Calendar
In this example we will assign Availability Only permission to a user on Room Mailbox. By assigning Availability Only permission, a user can see only the availability of the Room Mailbox.
Add-MailboxFolderPermission -Identity "Meeting-Room1:\Calendar" -User "ConceptsUser" -AccessRights AvailabilityOnly -Confirm:$false
Assign Limited Details permission on Room Mailbox Calendar
In this example we will assign Limited Details permission to a user on a Room Mailbox.
Add-MailboxFolderPermission -Identity "Meeting-Room1:\Calendar" -User "ConceptsUser" -AccessRights LimitedDetails -Confirm:$false
Assign Publishing Editor permission on Room Mailbox Calendar
In this example we will assign Publishing Editor permission to a user on a Room Mailbox.
Add-MailboxFolderPermission -Identity "Meeting-Room1:\Calendar" -User "ConceptsUser" -AccessRights PublishingEditor -Confirm:$false
Assign Reviewer permission on Room Mailbox calendar to each member of a group
In this example, we will assign Reviewer permission on a Room Mailbox to each member of the group.
# Specify the room mailbox and the group
$roomMailbox = "Meeting-Room5"
$group = "SecurityGroup"
# Get members of the group
$groupMembers = Get-DistributionGroupMember -Identity $group
# Retrieve the mailbox identity and construct the calendar identity
$mailboxIdentity = (Get-Mailbox -Identity $roomMailbox).Identity
$calendarIdentity = $mailboxIdentity + ":\Calendar"
# Loop through each member and assign "Reviewer" permission on the room mailbox calendar
foreach ($member in $groupMembers) {
Add-MailboxFolderPermission -Identity $calendarIdentity -User $member.PrimarySmtpAddress -AccessRights Reviewer -Confirm:$false
Write-Host "Reviewer permission assigned to $($member.PrimarySmtpAddress) on the calendar of $roomMailbox"
}
List Room Mailbox permissions using PowerShell
List users who have Full Access permission on Room Mailbox
Get-MailboxPermission -Identity "Meeting-Room5" | Select-Object Identity,User,AccessRights
The above PowerShell command will list all the users who have Full Access permission on a room mailbox as shown below:

Find Room Mailboxes on which a user has Full Access permission
In this example we will find the room mailboxes on which a user has Full Access permission.
Replace “Bob Ross” with the actual display name or email address of the user and run below PowerShell script:
# Specify the user for which you want to find room mailboxes with full access permission
$user = "Bob Ross"
# Get room mailboxes where the user has full access permission
$roomMailboxes = Get-Mailbox -RecipientTypeDetails RoomMailbox -ResultSize Unlimited | Where-Object {
Get-MailboxPermission -Identity $_.Identity -User $user -ErrorAction SilentlyContinue | Where-Object { $_.AccessRights -match "FullAccess" }
}
# Output the list of room mailboxes
$roomMailboxes
List users who have Send As permission on a Room Mailbox using PowerShell
In this example we will find users who have Send As permission on a room mailbox.
Get-RecipientPermission -Identity "meeting-room1" | Select-Object Identity,Trustee,AccessRights
List users who have Send on Behalf permission on a Room Mailbox using PowerShell
Get-Mailbox -Identity "Meeting-Room1" | Select-Object GrantSendonBehalfTo
Remove permissions for a Room Mailbox using PowerShell
Remove Full Access permission from a Room Mailbox
Remove-MailboxPermission -Identity "Meeting-Room1" -User "Bob Ross" -AccessRights FullAccess -InheritanceType All -Confirm:$false
Bulk remove Full Access permissions from Room Mailboxes using CSV and PowerShell
In this example we will remove Full Access permissions from Room Mailboxes using CSV and PowerShell.
Create a CSV file with below columns:
- Mailbox: Contains the name or email address of the room mailbox.
- User: Contains the name or email address of the user.
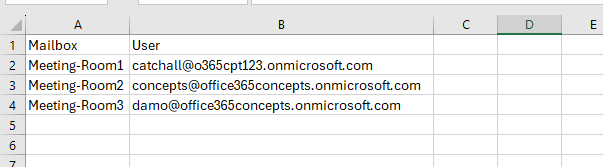
Replace “C:\CSV Files\bulkremovepermission.csv” with the actual path of your CSV file and run below PowerShell script:
# Path to the CSV file containing mailbox and user information
$csvFilePath = "C:\CSV Files\bulkremovepermission.csv"
# Import CSV file
$mailboxUsers = Import-Csv $csvFilePath
# Iterate through each entry in the CSV
foreach ($entry in $mailboxUsers) {
$mailbox = $entry.Mailbox
$userToRemove = $entry.User
# Remove Full Access permission from the user on the room mailbox
Remove-MailboxPermission -Identity $mailbox -User $userToRemove -AccessRights FullAccess -Confirm:$false
# Display output message
Write-Host "Full Access permission removed from $userToRemove on $mailbox"
}
Remove Send As permission for a Room Mailbox
Remove-RecipientPermission -Identity "Meeting-Room1" -Trustee "Test123" -AccessRights SendAs -Confirm:$false
Remove Send on Behalf permission for a Room Mailbox
Set-Mailbox -Identity "Meeting-Room1" -GrantSendOnBehalfTo $null
Conclusion
In this article you learnt how to manage Room Mailbox permissions using PowerShell commands. You learnt how to assign full access permission on a room mailbox using PowerShell, how to assign send as permission on a room mailbox, how to assign permission on room mailbox calendar using PowerShell, and how to remove these permissions.
If you found this article helpful and informative, please share it within your community and do not forget to share your feedback in the comments below.
You might like our other articles on Manage Distribution Groups using PowerShell, Manage Dynamic Distribution Groups using PowerShell, Manage Office 365 licenses using PowerShell, Manage Shared Mailboxes using PowerShell and Manage Room Mailboxes using PowerShell.
Please join us on YouTube for the latest videos on the Cloud technology and join our Newsletter for the early access of the articles and updates.
Happy Scripting!!