Manage Dynamic Distribution Groups using PowerShell
In this article you will learn how to manage Dynamic Distribution Groups using PowerShell commands.
Table of Contents
What is a Dynamic Distribution Group (DDG)
A Dynamic Distribution Group (DDG) is a type of distribution group in Exchange Online, whose membership is determined dynamically based on pre-defined criteria. Unlike Distribution Groups (DG) where members are added manually, a Dynamic Distribution Group automatically includes recipients based on the attributes such as their organization, department, location, or custom properties.
Manage Dynamic Distribution Groups using PowerShell
Using PowerShell to manage Dynamic Distribution Groups allows administrators to automate the creation, modification, and deletion of these groups, providing efficient and scalable management of email distribution lists. PowerShell cmdlets enable administrators to define the criteria for membership, configure filters, and update group properties as organizational requirements change.
Create Dynamic Distribution Groups
Connect to Exchange Online PowerShell
Before you run below commands, you need to connect to Exchange Online in Windows PowerShell using below command:
Connect-ExchangeOnline
Create Dynamic Distribution Group and add members basis on the Department attribute
The below script will create a dynamic distribution group and will add the User Mailboxes as member whose Department attribute is set to Sales.
# Define the criteria for the Dynamic Distribution Group
$GroupName = "SalesDDG1"
$GroupAlias = "SalesDDG1"
$GroupRecipientFilter = "Department -eq 'Sales' -and RecipientTypeDetails -eq 'UserMailbox'"
$GroupEmailAddress = "[email protected]" # Specify the desired email address
# Create the Dynamic Distribution Group
New-DynamicDistributionGroup -Name $GroupName -Alias $GroupAlias -RecipientFilter $GroupRecipientFilter
# Set the email address for the Dynamic Distribution Group
Set-DynamicDistributionGroup -Identity $GroupName -PrimarySmtpAddress $GroupEmailAddress
In the above script, modify $GroupName, $GroupAlias and $GroupEmailAddress as per your requirement.
Create Dynamic Distribution Group and add all the User Mailboxes as member
To create a Dynamic Distribution Group and add all the user mailboxes as member in group, run below PowerShell script:
# Define the criteria for the Dynamic Distribution Group
$GroupName = "DDG1"
$GroupAlias = "DDG1"
$GroupRecipientFilter = "RecipientTypeDetails -eq 'UserMailbox'"
$GroupEmailAddress = "[email protected]" # Specify the desired email address
# Create the Dynamic Distribution Group
New-DynamicDistributionGroup -Name $GroupName -Alias $GroupAlias -RecipientFilter $GroupRecipientFilter
# Set the email address for the Dynamic Distribution Group
Set-DynamicDistributionGroup -Identity $GroupName -PrimarySmtpAddress $GroupEmailAddress
In the above script, modify $GroupName, $GroupAlias and $GroupEmailAddress as per your requirement.
Exclude shared mailbox in Dynamic Distribution Group membership
In this example we will create a dynamic distribution group, we will add all the mailboxes as members but we will exclude shared mailboxes.
# Define the criteria for the Dynamic Distribution Group
$GroupName = "DDG3"
$GroupAlias = "DDG3"
$GroupRecipientFilter = "(RecipientType -eq 'UserMailbox' -or RecipientType -eq 'MailUser') -and RecipientTypeDetails -ne 'SharedMailbox'"
$GroupEmailAddress = "[email protected]" # Specify the desired email address
# Create the Dynamic Distribution Group
New-DynamicDistributionGroup -Name $GroupName -Alias $GroupAlias -RecipientFilter $GroupRecipientFilter
# Set the email address for the Dynamic Distribution Group
Set-DynamicDistributionGroup -Identity $GroupName -PrimarySmtpAddress $GroupEmailAddress
In the above script, modify $GroupName, $GroupAlias and $GroupEmailAddress as per your requirement.
Create Dynamic Distribution Group and add resource mailboxes as member
If your requirement is to create a dynamic distribution group and you want to add only resource mailboxes as member, run below PowerShell script:
# Define the criteria for the Dynamic Distribution Group
$GroupName = "DDG4"
$GroupAlias = "DDG4"
$GroupRecipientFilter = "RecipientType -eq 'UserMailbox' -and RecipientTypeDetails -eq 'RoomMailbox' -or RecipientTypeDetails -eq 'EquipmentMailbox'"
$GroupEmailAddress = "[email protected]" # Specify the desired email address
# Create the Dynamic Distribution Group
New-DynamicDistributionGroup -Name $GroupName -Alias $GroupAlias -RecipientFilter $GroupRecipientFilter
# Set the email address for the Dynamic Distribution Group
Set-DynamicDistributionGroup -Identity $GroupName -PrimarySmtpAddress $GroupEmailAddress
In the above script, modify $GroupName, $GroupAlias and $GroupEmailAddress as per your requirement.
Bulk create Dynamic Distribution Group in Office 365
In this scenario we will create bulk Dynamic Distribution Groups using a CSV file. We will add members in all these DDGs basis on the CustomAttribute1 of the User Mailboxes.
Create CSV file: We will create a CSV file with below values:
Name: Represents the name of the Dynamic Distribution Group.
EmailAddress: Contains the primary email address for each DDG.
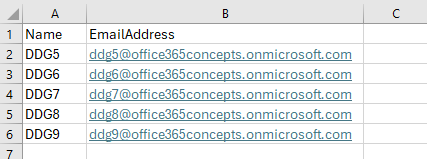
Run Script: The below script will create create the dynamic distribution groups basis on the Name and PrimarySmtpAddress attributes within the CSV file, and it will add the User Mailboxes whose CustomAttrribute1 is set to Sales.
# Function to create Dynamic Distribution Group
Function Create-DDG {
param(
[string]$Name,
[string]$EmailAddress,
[string]$RecipientFilter
)
# Create the Dynamic Distribution Group
New-DynamicDistributionGroup -Name $Name -Alias $Name -PrimarySmtpAddress $EmailAddress -RecipientFilter $RecipientFilter
}
# Path to CSV file
$CSVPath = "C:\CSV Files\createddg.csv"
# Recipient filter
$RecipientFilter = "((RecipientType -eq 'UserMailbox') -and (CustomAttribute1 -eq 'sales'))"
# Import CSV and loop through each entry
$CSVData = Import-Csv $CSVPath
foreach ($Entry in $CSVData) {
# Extract data from CSV
$Name = $Entry.Name
$EmailAddress = $Entry.EmailAddress
# Create Dynamic Distribution Group
Create-DDG -Name $Name -EmailAddress $EmailAddress -RecipientFilter $RecipientFilter
}
View membership of Dynamic Distribution groups
Refresh Dynamic Distribution Group
If a dynamic distribution group is not displaying its members, you can refresh the dynamic distribution group by running below PowerShell command:
Set-DynamicDistributionGroup -Identity "DDG Name" -ForceMembershipRefresh
Get Dynamic Distribution Group members
If you want to list the members of a dynamic distribution group, run below PowerShell command:
Get-DynamicDistributionGroupMember -Identity "DDG5" | Select-Object DisplayName, PrimarySMTPAddress
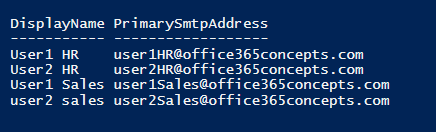
Get members of all Dynamic Distribution Groups
If you want to list members of all the dynamic distribution groups, run below PowerShell script:
# Get all Dynamic Distribution Groups
$DDGs = Get-DynamicDistributionGroup -Filter {RecipientTypeDetails -eq 'DynamicDistributionGroup'}
# Loop through each DDG and get its members
foreach ($DDG in $DDGs) {
Write-Host "DDG Name: $($DDG.Name)"
Write-Host "Members:"
$Members = Get-Recipient -RecipientPreviewFilter $DDG.RecipientFilter | Select-Object DisplayName,PrimarySmtpAddress
foreach ($Member in $Members) {
Write-Host " $($Member.DisplayName) - $($Member.PrimarySmtpAddress)"
}
Write-Host "==============================="
}
The above list will display all the members of all dynamic distribution groups in Exchange Online organization as shown below:
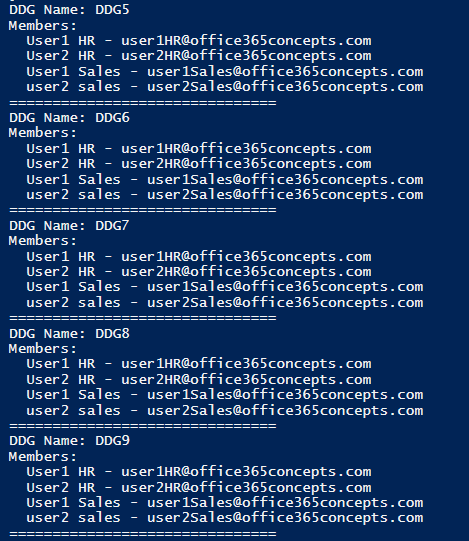
Export Dynamic Distribution Group members to csv
If you want to list members of all the dynamic distribution groups and export them to CSV file, run below PowerShell script:
# Get all Dynamic Distribution Groups
$DDGs = Get-DynamicDistributionGroup -Filter {RecipientTypeDetails -eq 'DynamicDistributionGroup'}
# Initialize an array to store the results
$Results = @()
# Loop through each DDG and get its members
foreach ($DDG in $DDGs) {
$Members = Get-Recipient -RecipientPreviewFilter $DDG.RecipientFilter | Select-Object DisplayName,PrimarySmtpAddress | ForEach-Object { "$($_.DisplayName) <$($_.PrimarySmtpAddress)>" }
# Add the DDG name and its members to the results array
$Results += [PSCustomObject]@{
"DDG Name" = $DDG.Name
"Members" = $Members -join ", "
}
}
# Export the results to a CSV file
$Results | Export-Csv -Path "C:\CSV Files\DDG_Members1.csv" -NoTypeInformation
The above script will export members of all the dynamic distribution groups in a CSV file as shown below:
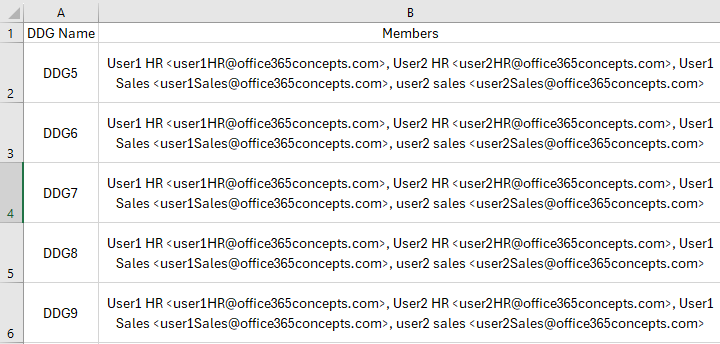
View owners of all Dynamic Distribution Groups
To list owners of all the dynamic distribution groups, run below PowerShell script:
# Get all Dynamic Distribution Groups
$DDGs = Get-DynamicDistributionGroup -ResultSize Unlimited
# Loop through each DDG and list its owners
foreach ($DDG in $DDGs) {
$Owners = Get-DynamicDistributionGroup -Identity $DDG.DistinguishedName | Select-Object -ExpandProperty ManagedBy
$OwnerNames = @()
foreach ($Owner in $Owners) {
$OwnerName = (Get-Recipient $Owner).DisplayName
$OwnerNames += $OwnerName
}
$OwnersString = $OwnerNames -join ', '
Write-Host "DDG Name: $($DDG.Name)"
Write-Host "Owners: $($OwnersString)"
Write-Host "==============================="
}
The above script will display the name of each DDG and its owner as shown below:
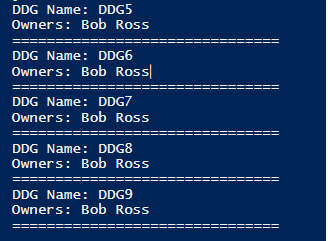
Add Owner to Dynamic Distribution Groups
Add owner in Dynamic Distribution Group
To add owner in a dynamic distribution group, run below PowerShell command:
Set-DynamicDistributionGroup -Identity "DDG5" -ManagedBy "Bob Ross"
Add a user as owner in all Dynamic Distribution Groups
If you want to add a user as owner in all the dynamic distribution groups, run below PowerShell command:
Get-DynamicDistributionGroup -ResultSize Unlimited | Set-DynamicDistributionGroup -ManagedBy "Bob Ross"
Add owners in Dynamic Distribution Groups using CSV file
If you want to add owners in dynamic distribution groups using a CSV file, create a CSV file with below values:
Name: This is the name of each DDG.
OwnerDisplayName: This is the display name of each owner you want to add in DDG.
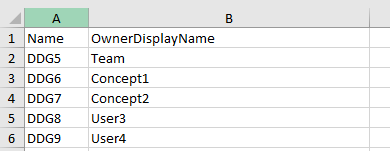
Run below PowerShell script:
# Function to add owners to Dynamic Distribution Group
Function Add-OwnersToDDG {
param(
[string]$GroupName,
[string]$OwnerEmailAddress
)
# Get existing owners of the Dynamic Distribution Group
$DDG = Get-DynamicDistributionGroup -Identity $GroupName
$ExistingOwners = $DDG.ManagedBy
# Add the new owner to the existing owners
$NewOwners = $ExistingOwners + $OwnerEmailAddress
# Set the owners of the Dynamic Distribution Group
Set-DynamicDistributionGroup -Identity $GroupName -ManagedBy $NewOwners
}
# Path to CSV file containing DDG owners information (Name, OwnerEmailAddress)
$CSVPath = "C:\CSV Files\DDG_Owners.csv"
# Import CSV and loop through each entry
$CSVData = Import-Csv $CSVPath
foreach ($Entry in $CSVData) {
# Extract data from CSV
$GroupName = $Entry.Name
$OwnerEmailAddress = $Entry.OwnerEmailAddress
# Add owner to the Dynamic Distribution Group
Add-OwnersToDDG -GroupName $GroupName -OwnerEmailAddress $OwnerEmailAddress
}
Modify membership of Dynamic Distribution Groups
Add members in Dynamic Distribution Group basis on CustomAttribute1
If you want to modify the membership of a dynamic distribution group, run below PowerShell script:
# Set the recipient filter
$RecipientFilter = "((RecipientType -eq 'UserMailbox' -or RecipientType -eq 'MailUniversalDistributionGroup' -or RecipientType -eq 'MailUniversalSecurityGroup' -or RecipientType -eq 'MailNonUniversalGroup') -and (CustomAttribute1 -eq 'Sales'))"
# Get the Dynamic Distribution Group
$DDG = Get-DynamicDistributionGroup -Identity "DDG5"
# Set the recipient filter for the DDG
Set-DynamicDistributionGroup -Identity $DDG.Identity -RecipientFilter $RecipientFilter
Replace “YourDDGName” with the name or identity of your Dynamic Distribution Group (DDG).
This script sets the recipient filter for the DDG to include all user mailboxes, groups (both universal and non-universal), and mail users with the custom attribute CustomAttribute1 set to ‘Sales’.
Add members in Dynamic Distribution Group basis on CustomAttribute1 and City attributes
The below script will modify the membership of dynamic distribution group, and will add the members whose City attribute is set to Delhi and CustomAttribute1 is set to Test.
# Set the recipient filter
$RecipientFilter = "((RecipientType -eq 'UserMailbox' -or RecipientType -eq 'MailUniversalDistributionGroup' -or RecipientType -eq 'MailUniversalSecurityGroup' -or RecipientType -eq 'MailNonUniversalGroup') -and (City -eq 'Delhi') -and (CustomAttribute1 -eq 'Test'))"
# Get the Dynamic Distribution Group
$DDG = Get-DynamicDistributionGroup -Identity "DDG9"
# Set the recipient filter for the DDG
Set-DynamicDistributionGroup -Identity $DDG.Identity -RecipientFilter $RecipientFilter
Replace “YourDDGName” with the name or identity of your Dynamic Distribution Group (DDG).
This script sets the recipient filter for the DDG to include all user mailboxes, groups (both universal and non-universal), and mail users with the City attribute set to ‘Delhi‘ and the CustomAttribute1 set to ‘Test’.
Manage Dynamic Distribution Group permissions
Add Send As permission on Dynamic Distribution Group
To assign a user Send As permission on a dynamic distribution group, run below PowerShell command:
Add-RecipientPermission -Identity "DDG6" -Trustee "Bob Ross" -AccessRights SendAs
To verify if permission is added, run below PowerShell command:
Get-RecipientPermission -Identity "DDG6"
In the below image you can see the permission has been granted to user “Bob Ross” on dynamic distribution group “DDG6”.

Add Send on Behalf permission on Dynamic Distribution Group
If you want to assign a user Send on Behalf permission on a dynamic distribution group, run below PowerShell command:
Set-DynamicDistributionGroup -Identity "DDG6" -GrantSendOnBehalfTo "User2 HR"
The above command will assign Send on Behalf permission to “User2 HR” on dynamic distribution group “DDG6”.
To verify the permission, run below PowerShell command:
Get-DynamicDistributionGroup -Identity "DDG6" | Select-Object GrantSendonBehalfTo
Manage Dynamic Distribution Group email delivery properties
Set Dynamic Distribution Group to accept emails from outside organization
If a dynamic distribution group is not accepting emails from outside the organization (external emails), run below command to verify RequireSenderAuthenticationEnabled attribute:
Get-DynamicDistributionGroup -Identity "DDG5" | Select-Object RequireSenderAuthenticationEnabled
The RequireSenderAuthenticationEnabled parameter specifies whether a dynamic distribution group should receive emails from external senders or not. By default this parameter is set to True, that indicates the DDG is set to receive the emails only from internal senders, and the emails sent from external senders will be rejected.
To allow a dynamic distribution group to receive emails from both internal (authenticated) and external users, run below PowerShell command:
Set-DynamicDistributionGroup -Identity "DDG5" -RequireSenderAuthenticationEnabled $false
The value $false indicates the the dynamic distribution group is allowed to receive emails from both authenticated (internal) and external senders.
Set Dynamic Distribution Group to receive emails only from specific senders
If you want to allow a dynamic distribution group to receive emails only from specific senders, run below PowerShell command:
Set-DynamicDistributionGroup -Identity "DDG6" -AcceptMessagesOnlyFrom "User1 HR","User2 HR"
To verify, please run below PowerShell command:
Get-DynamicDistributionGroup -Identity "DDG6" | Select-Object AcceptMessagesOnlyFrom
Set Dynamic Distribution Group to receive emails only from the members of specific DLs
If you want to allow a dynamic distribution group to receive emails only from the members of specific distribution groups, run below PowerShell command:
Set-DynamicDistributionGroup -Identity "DDG6" -AcceptMessagesOnlyFromDLMembers DG1,DG2
Where DG1 and DG2 are the names of distribution groups. Specifying a group means all members of the group are allowed to send messages to this recipient.
To verify the changes, run below PowerShell command:
Get-DynamicDistributionGroup -Identity "DDG6" | Select-Object AcceptMessagesOnlyFromDLMembers
Conclusion
In this article you learnt how to manage Dynamic Distribution Groups using PowerShell, you learnt how to refresh dynamic distribution group if it doesn’t display membership, you learnt how to view membership of dynamic distribution groups, how to bulk create dynamic distribution group in Office 365, and how to manage dynamic distribution group email delivery properties.
You might like our other article on Manage Distribution Groups using PowerShell.
If you found this article helpful and informative, please share it within your community and do not forget to share your feedback in the comments below. Please join us on our YouTube channel for the latest videos on Cloud technology and join our Newsletter for the early access of the articles and updates.
Happy Scripting!!