Get mailbox folder size using PowerShell
In this article we will learn how to get mailbox folder size using PowerShell commands. We will discuss all the possible scenarios where we can get folder size of mailboxes in Exchange Online. We will learn how to get mailbox folder size for single mailbox, for multiple mailboxes, we will filter all the folders and get their size exported to CSV file, and so on.
Table of Contents
Get mailbox folder size using PowerShell
With PowerShell, administrators can automate the process of retrieving mailbox folder statistics across multiple users, allowing for streamlined monitoring and management of mailbox storage usage.
“Get-MailboxFolderStatistics” is a PowerShell cmdlet used in Exchange Online environments to retrieve detailed information about mailbox folders’ sizes. This cmdlet enables administrators to assess the storage usage of individual folders within a user’s mailbox, facilitating effective management of storage resources.
Connect to Exchange Online PowerShell
To be able to run below PowerShell commands, you need to connect to Exchange Online PowerShell module. Open Windows PowerShell as administrator and run below commands one by one:
Install-Module ExchangeOnlineManagement
Import-Module ExchangeOnlineManagement
Connect-ExchangeOnline
Get mailbox folder size for 1 mailbox
In this example, we will get mailbox folder size of 1 mailbox. Replace “Concepts User” with the actual name of the mailbox, and run below PowerShell command:
Get-MailboxFolderStatistics -Identity "Concepts User" | Select-Object Name,FolderType,FolderSize
The above PowerShell command will display the Folder Name along with the Size of each folder of “Concepts User” mailbox as shown below:
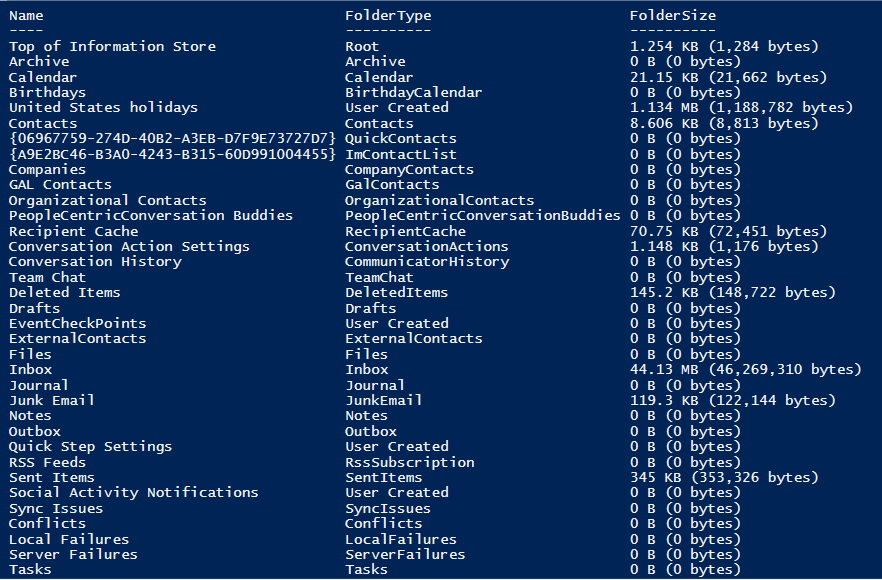
Get size of default folders of a mailbox
In this example we will filter the default folders of a mailbox, and will get the size of each folder. Replace “Concepts User” with the actual name of the mailbox and run below PowerShell script.
# Define the default folder names
$defaultFolders = @("Inbox", "SentItems", "DeletedItems", "JunkEmail", "Drafts")
# Iterate through each default folder and retrieve its statistics
foreach ($folder in $defaultFolders) {
Get-MailboxFolderStatistics -Identity "Concepts User" -FolderScope $folder | Select-Object Name,FolderType,FolderSize
}
The above PowerShell script will display the size of each default folder of “Concepts User” mailbox as shown below:
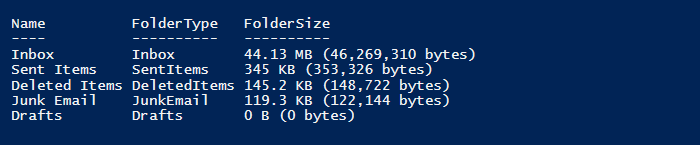
Get size of Inbox folder for a mailbox
In this example, we will retrieve size of Inbox folder of a mailbox. Replace “Concepts user” with the actual name of the mailbox and run below PowerShell command:
# Define the default folder names
$foldername = @("Inbox")
# Iterate through each default folder and retrieve its statistics
foreach ($folder in $foldername) {
Get-MailboxFolderStatistics -Identity "Concepts User" -FolderScope $folder | Select-Object Name,FolderPath, FolderSize
}
Get size of folders of a mailbox excluding default folders
In this example, we will get size of all folders of a mailbox excluding the default folders (Inbox, Deleted Items, Sent Items, Junk Email, and Drafts). Replace “Concepts user” with the actual name of the mailbox and run below PowerShell script.
# Define the default folder names
$defaultFolders = @("Inbox", "Sent Items", "Deleted Items", "Junk Email", "Drafts")
# Get mailbox folder statistics excluding default folders
Get-MailboxFolderStatistics -Identity "Concepts User" |
Where-Object { $defaultFolders -notcontains $_.FolderPath.Split("/")[-1] } |
Select-Object FolderPath, FolderSize,Name
Get mailbox folder size of all mailboxes using PowerShell
In this example we will retrieve folder size of all mailboxes in Exchange Online tenant.
# Get the folder size of all mailboxes
$mailboxes = Get-Mailbox -ResultSize Unlimited
foreach ($mailbox in $mailboxes) {
$folderStats = Get-MailboxFolderStatistics -Identity $mailbox.Identity
foreach ($folderStat in $folderStats) {
Write-Host "Mailbox: $($mailbox.DisplayName), Folder: $($folderStat.FolderPath), Size: $($folderStat.FolderAndSubfolderSize)"
}
}
Get mailbox folder size of multiple mailboxes using CSV file
In this example we will use CSV file and PowerShell to get folder size of multiple mailboxes.
Create a CSV file with a column PrimarySmtpAddress that will contain the email address of each user for whom you want to get folders size.
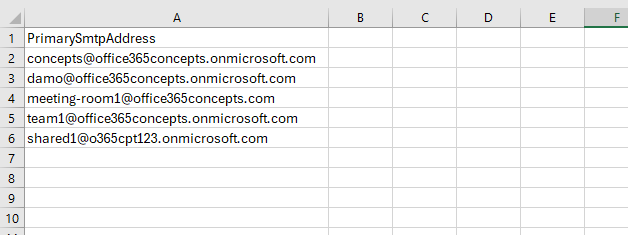
Replace “C:\CSV Files\foldersize.csv” with the actual path of your CSV file and run below PowerShell script:
# Specify the path to the CSV file
$csvFilePath = "C:\CSV Files\foldersize.csv"
# Read the CSV file
$mailboxes = Import-Csv -Path $csvFilePath
foreach ($mailbox in $mailboxes) {
$mailboxIdentity = $mailbox.PrimarySMTPAddress
$folderStats = Get-MailboxFolderStatistics -Identity $mailboxIdentity
foreach ($folderStat in $folderStats) {
Write-Host "Mailbox: $($mailboxIdentity), Folder: $($folderStat.FolderPath), Size: $($folderStat.FolderAndSubfolderSize)"
}
}
If you want to export the output to a CSV file instead of displaying it on PowerShell console, use below PowerShell script:
# Specify the path to the CSV file
$csvFilePath = "C:\CSV Files\foldersize.csv"
# Read the CSV file
$mailboxes = Import-Csv -Path $csvFilePath
# Create an empty array to store folder statistics
$folderStatsArray = @()
foreach ($mailbox in $mailboxes) {
$mailboxIdentity = $mailbox.PrimarySMTPAddress
$folderStats = Get-MailboxFolderStatistics -Identity $mailboxIdentity
foreach ($folderStat in $folderStats) {
$folderStatsObject = [PSCustomObject]@{
"Mailbox" = $mailboxIdentity
"Folder" = $folderStat.FolderPath
"Size" = $folderStat.FolderAndSubfolderSize
}
$folderStatsArray += $folderStatsObject
}
}
# Export the folder statistics to a CSV file
$folderStatsArray | Export-Csv -Path "C:\CSV Files\FolderStatistics.csv" -NoTypeInformation
The above script will read the CSV file from the path “C:\CSV Files\foldersize.csv“, will retrieve the folders size of the mailboxes mentioned in the CSV file, and will export their folder size in FolderStatistics.csv file. Replace CSV file with the actual path of your CSV files.
Get folder size of all mailboxes and export to CSV file
In this example we will retrieve the folder size of all mailboxes and export the output to CSV file. Replace “C:\CSV Files\AllMailboxFolderStatistics.csv” with the actual path where you want to save CSV file, and run below PowerShell script:
# Get all mailboxes in the Exchange Online tenant
$mailboxes = Get-Mailbox -ResultSize Unlimited
# Create an empty array to store folder statistics
$folderStatsArray = @()
foreach ($mailbox in $mailboxes) {
$mailboxIdentity = $mailbox.Identity
$folderStats = Get-MailboxFolderStatistics -Identity $mailboxIdentity
foreach ($folderStat in $folderStats) {
$folderStatsObject = [PSCustomObject]@{
"Mailbox" = $mailboxIdentity
"Folder" = $folderStat.FolderPath
"Size" = $folderStat.FolderAndSubfolderSize
}
$folderStatsArray += $folderStatsObject
}
}
# Export the folder statistics to a CSV file
$folderStatsArray | Export-Csv -Path "C:\CSV Files\AllMailboxFolderStatistics.csv" -NoTypeInformation
Get size of Calendar folder of a mailbox
In this example we will retrieve size of Calendar folder of a mailbox. Replace “Concepts User” with the actual display name or email address of the mailbox and run below PowerShell command:
# Define the default folder names
$defaultFolders = @("Calendar")
# Iterate through each default folder and retrieve its statistics
foreach ($folder in $defaultFolders) {
Get-MailboxFolderStatistics -Identity "Concepts User" -FolderScope $folder | Select-Object Name,FolderType,FolderSize
}
The above command will display the size of all calendars of a mailbox as shown below:

Get size of Calendar folder for all mailboxes
In this example we will retrieve size of Calendar folder for all mailboxes.
# Get all mailboxes in the Exchange Online tenant
$mailboxes = Get-Mailbox -ResultSize Unlimited
# Define the default folder names
$defaultFolders = @("Calendar")
# Create an empty array to store folder statistics
$folderStatsArray = @()
# Iterate through each mailbox
foreach ($mailbox in $mailboxes) {
# Check if the mailbox identity is not null
if ($mailbox.Identity) {
# Iterate through each default folder for the mailbox
foreach ($folder in $defaultFolders) {
# Retrieve folder statistics for the default folder
$folderStats = Get-MailboxFolderStatistics -Identity $mailbox.Identity -FolderScope $folder -ErrorAction SilentlyContinue
# Check if folder statistics are retrieved successfully
if ($folderStats) {
foreach ($stats in $folderStats) {
$folderStatsObject = [PSCustomObject]@{
"MailboxDisplayName" = $mailbox.DisplayName
"MailboxEmailAddress" = $mailbox.PrimarySmtpAddress
"FolderName" = $stats.Name
"FolderType" = $stats.FolderType
"FolderSize" = $stats.FolderAndSubfolderSize
}
$folderStatsArray += $folderStatsObject
}
}
}
}
}
# Display the folder statistics
$folderStatsArray
Get size of Contacts folder of a mailbox
In this example we will retrieve size of Contacts folder of a mailbox. Replace “Concepts User” with the actual display name or email address of the mailbox and run below PowerShell command:
# Define the default folder names
$defaultFolders = @("Contacts")
# Iterate through each default folder and retrieve its statistics
foreach ($folder in $defaultFolders) {
Get-MailboxFolderStatistics -Identity "Concepts User" -FolderScope $folder | Select-Object Name,FolderType,FolderSize
}
Get size of subfolders of Inbox folder of a mailbox
In this example we will retrieve size of subfolders of Inbox folder of a mailbox. Replace “Concepts User” with the actual display name of the mailbox and run below PowerShell script:
# Specify the user's email address
$userEmailAddress = "Concepts User"
# Get the size of all subfolders within the Inbox for the user
$inboxSubfolderStats = Get-MailboxFolderStatistics -Identity $userEmailAddress -FolderScope Inbox
# Filter out only the subfolders
$subfolders = $inboxSubfolderStats | Where-Object { $_.FolderPath -ne '\' }
# Display the size of each subfolder within the Inbox
$subfolders | Select-Object FolderPath, FolderAndSubfolderSize
The above PowerShell script will display the subfolders of Inbox folder and their size as shown below:
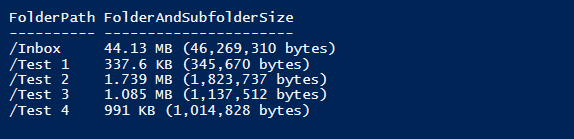
Get size of subfolders of Inbox folder of all mailboxes
In this example we will retrieve size of subfolders of Inbox folder of all the mailboxes.
# Get all mailboxes in the Exchange Online tenant
$mailboxes = Get-Mailbox -ResultSize Unlimited
# Create an empty array to store subfolder statistics
$subfolderStatsArray = @()
# Iterate through each mailbox
foreach ($mailbox in $mailboxes) {
# Get the size of all subfolders within the Inbox for the current mailbox
$inboxSubfolderStats = Get-MailboxFolderStatistics -Identity $mailbox.Identity -FolderScope Inbox -ErrorAction SilentlyContinue
# Check if subfolder statistics are retrieved successfully
if ($inboxSubfolderStats) {
# Filter out only the subfolders
$subfolders = $inboxSubfolderStats | Where-Object { $_.FolderPath -ne '\' }
# Add subfolder statistics for the current mailbox to the main array
$subfolderStatsArray += $subfolders | Select-Object @{Name="MailboxDisplayName";Expression={$mailbox.DisplayName}}, FolderPath, FolderAndSubfolderSize
}
}
# Display the subfolder statistics
$subfolderStatsArray
Get empty folders of a mailbox
In this example, we will retrieve empty folders of a mailbox. Replace “Concepts User” with the actual display name of the mailbox and run below PowerShell script:
# Specify the user's email address
$userEmailAddress = "Concepts User"
# Get the folder statistics for all folders in the user's mailbox
$mailboxFolderStats = Get-MailboxFolderStatistics -Identity $userEmailAddress
# Initialize an array to store empty folders
$emptyFolders = @()
# Iterate through each folder statistics
foreach ($folderStat in $mailboxFolderStats) {
# Check if the folder is empty
if ($folderStat.ItemsInFolder -eq 0) {
$emptyFolders += $folderStat.Name
}
}
# Display empty folders
$emptyFolders
Conclusion
In this article you learnt how to get mailbox folder size using PowerShell. You might like our other articles on Get mailbox size report using PowerShell and Get Exchange Online mailbox size in GB.
If you found this article helpful and informative, please share it within your community and do not forget to share your feedback in the comments below.
Join us on YouTube for the latest videos on the Cloud technology and join our Newsletter for the early access of the articles and updates.
Happy Scripting!!
I loved this so much. Seems it would help me a lot in future.
Thank you for all of your informative blogs😊 🙏
Hi Avirup,
Thanks a lot!
You might like: Get mailbox size in GB.
Happy Scripting!!