Office 365 mailbox size report
In this article we will learn how to use PowerShell commands to get Office 365 mailbox size report.
Table of Contents
Standard mailbox size in Exchange Online
The standard mailbox size in Exchange Online depends on the license you assign to the user account. Microsoft 365 licenses have Exchange Online Plan 1 and Exchange Online Plan 2 included. If a user account has Exchange Online Plan 1 license assigned, he will get a mailbox assigned with 50GB storage space. Whereas, the user account that has Exchange Online Plan 2 license assigned, will get 100GB storage space.
Shared Mailbox size limit in Exchange Online
By default a shared mailbox size limit is 50GB. When an administrator creates a shared mailbox either from Exchange Admin Center or PowerShell, it gets a storage space of 50GB. However, we can increase the shared mailbox size limit to 100GB by assigning it Exchange Online Plan 2 license.
Office 365 mailbox size report
Now let’s learn how to get Office 365 mailbox size report. We will use PowerShell commands to prepare Exchange Online mailbox size report for single user and multiple users. We will also see how to get folder level storage report.
Connect to Exchange Online PowerShell
To be able to run below PowerShell commands, you need to connect to Exchange Online PowerShell. Open Windows PowerShell as administrator and run below commands one by one:
Install-Module ExchangeOnlineManagement
Import-Module ExchangeOnlineManagement
Connect-ExchangeOnline
Get mailbox size of 1 user using PowerShell
In this example we will find the mailbox size for 1 user. Replace “Concepts User” with the actual name of the user and run below PowerShell command:
Get-Mailbox -Identity "Concepts User" | Get-MailboxStatistics | Select-Object ItemCount,TotalItemSize
The above command will display the total size of items in a mailbox along with the count as shown below:

Get mailbox size for all mailboxes using PowerShell
In this example we will get mailbox size for all the mailboxes.
Get-Mailbox -ResultSize Unlimited | Get-MailboxStatistics | Select-Object DisplayName,ItemCount,TotalItemSize
Get mailbox size for all mailboxes and export to CSV file
In this example we will export mailbox size for all mailboxes in Exchange Online organization. Replace “C:\CSV Files\MailboxSize.csv” with the actual CSV file path and run below PowerShell command:
# Get all user mailboxes and their sizes
$mailboxSizes = Get-Mailbox -ResultSize Unlimited | Get-MailboxStatistics | Select-Object DisplayName,ItemCount,TotalItemSize
# Export mailbox sizes to a CSV file
$mailboxSizes | Export-Csv -Path "C:\CSV Files\MailboxSize.csv" -NoTypeInformation
The above command will fetch mailbox size for all mailboxes and export the output in a CSV file as shown below:
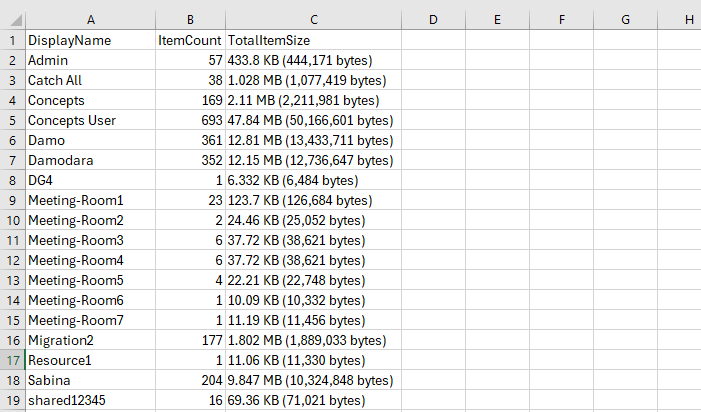
Get mailbox size for inactive mailboxes
In this example we will get mailbox size report of all inactive mailboxes.
Get-Mailbox -InactiveMailboxOnly -ResultSize Unlimited | Get-MailboxStatistics | Select-Object DisplayName,ItemCount,TotalItemSize
Get mailbox size for all shared mailboxes
In this example, we will get mailbox size report for all shared mailboxes.
Get-Mailbox -RecipientTypeDetails "SharedMailbox" -ResultSize Unlimited | Get-MailboxStatistics | Select-Object DisplayName,ItemCount,TotalItemSize
Get mailbox size for all room mailboxes
In this example, we will get mailbox size report for all room mailboxes.
Get-Mailbox -RecipientTypeDetails "RoomMailbox" -ResultSize Unlimited | Get-MailboxStatistics | Select-Object DisplayName,ItemCount,TotalItemSize
Get top 10 mailbox with largest mailbox size
In this example we will use PowerShell to find top 10 mailboxes that have largest mailbox size.
# Get all user mailboxes and their sizes
$mailboxes = Get-Mailbox -ResultSize Unlimited
$mailboxSizes = foreach ($mailbox in $mailboxes) {
$mailboxStatistics = Get-MailboxStatistics -Identity $mailbox.PrimarySmtpAddress -ErrorAction SilentlyContinue
if ($mailboxStatistics -ne $null) {
[PSCustomObject]@{
DisplayName = $mailbox.DisplayName
PrimarySmtpAddress = $mailbox.PrimarySmtpAddress
Size = $mailboxStatistics.TotalItemSize
}
}
}
# Sort mailbox sizes in descending order and select top 10
$top10Mailboxes = $mailboxSizes | Sort-Object -Property Size -Descending | Select-Object -First 10
# Display top 10 mailboxes with largest sizes
$top10Mailboxes | Format-Table -AutoSize
Get top 50 mailbox with largest mailbox size
In this example we will use PowerShell to find top 50 mailboxes that have largest mailbox size.
# Get all user mailboxes and their sizes
$mailboxes = Get-Mailbox -ResultSize Unlimited
$mailboxSizes = foreach ($mailbox in $mailboxes) {
$mailboxStatistics = Get-MailboxStatistics -Identity $mailbox.PrimarySmtpAddress -ErrorAction SilentlyContinue
if ($mailboxStatistics -ne $null) {
[PSCustomObject]@{
DisplayName = $mailbox.DisplayName
PrimarySmtpAddress = $mailbox.PrimarySmtpAddress
Size = $mailboxStatistics.TotalItemSize
}
}
}
# Sort mailbox sizes in descending order and select top 50
$top10Mailboxes = $mailboxSizes | Sort-Object -Property Size -Descending | Select-Object -First 50
# Display top 50 mailboxes with largest sizes
$top10Mailboxes | Format-Table -AutoSize
Find mailboxes with mailbox size larger than 10MB
In this example we will find the mailboxes that have mailbox size larger than 10MB.
Get-Mailbox | Get-MailboxStatistics | Where-Object {
$totalItemSize = $_.TotalItemSize
if ($totalItemSize -match '(\d+(\.\d+)?)\s*(KB|MB|GB)') {
$size = [double]$matches[1]
$unit = $matches[3]
switch ($unit) {
'KB' { $size = $size / 1KB }
'MB' { $size = $size }
'GB' { $size = $size * 1KB }
}
if ($size -gt 10) {
[PSCustomObject]@{
DisplayName = $_.DisplayName
TotalItemSize = $_.TotalItemSize
ItemCount = $_.ItemCount
}
}
}
} | Format-Table -AutoSize
Find mailboxes with mailbox size larger than 40GB
In this example we will find mailboxes that have mailbox size larger than 40GB.
$mailboxes = Get-Mailbox | Get-MailboxStatistics | Where-Object {
$totalItemSize = $_.TotalItemSize
if ($totalItemSize -match '(\d+(\.\d+)?)\s*(KB|MB|GB)') {
$size = [double]$matches[1]
$unit = $matches[3]
switch ($unit) {
'KB' { $size = $size / 1KB }
'MB' { $size = $size / 1MB }
'GB' { $size = $size }
}
if ($size -gt 40) {
[PSCustomObject]@{
DisplayName = $_.DisplayName
TotalItemSize = $_.TotalItemSize
ItemCount = $_.ItemCount
}
}
}
}
if ($mailboxes.Count -eq 0) {
Write-Host "No mailboxes found with a size larger than 40 GB."
} else {
$mailboxes | Format-Table -AutoSize
}
Conclusion
In this article you learnt how to get Office 365 mailbox size report using PowerShell. You might like our other articles on Restore inactive mailbox in Exchange Online and Manage user mailbox using PowerShell.
If you found this article helpful and informative, please share it within your community and do not forget to share your feedback in the comments below.
Join us on YouTube for the latest videos on the Cloud technology and join our Newsletter for the early access of the articles and updates.
Happy Scripting!!