Remove users access from Shared Mailbox in Bulk
In this blog we will learn how to use PowerShell commands to find what Shared Mailboxes a user is member of, we remove user access from Shared Mailbox, and we will also use PowerShell commands to bulk remove access from all Shared Mailboxes.
Table of Contents
Find what shared mailboxes a user is a member of
In this scenario we will use PowerShell script to find all the shared mailboxes a user is a member of. We will list the display name and email address of the shared mailboxes to which a user has Full Access permissions assigned.
Replace [email protected] with the actual email address of the user and run below PowerShell script.
# Define the user's email address
$userEmailAddress = "[email protected]"
# Get all shared mailboxes
$sharedMailboxes = Get-Mailbox -RecipientTypeDetails SharedMailbox
# Initialize an array to store results
$results = @()
# Iterate through each shared mailbox
foreach ($mailbox in $sharedMailboxes) {
# Check if the user has full access to the shared mailbox
$fullAccessPermissions = Get-MailboxPermission $mailbox.Identity | Where-Object { $_.AccessRights -match "FullAccess" -and $_.User -like "*$userEmailAddress*" }
# If user has full access, add the mailbox to results
if ($fullAccessPermissions) {
$displayName = $mailbox.DisplayName
$emailAddress = $mailbox.PrimarySmtpAddress
$results += [PSCustomObject]@{
DisplayName = $displayName
EmailAddress = $emailAddress
}
}
}
# Display the results
$results
The above PowerShell script will display the list of shared mailboxes on which [email protected] has Full Access permission as shown below:

Remove user access from shared mailbox in bulk
In this example we will use PowerShell to remove Full Access permission for a user from all shared mailboxes.
Replace [email protected] with the actual email address of the user for whom you want to remove Full Access permission, and run below PowerShell script:
# Define the user's email address
$userEmailAddress = "[email protected]"
# Get all shared mailboxes
$sharedMailboxes = Get-Mailbox -RecipientTypeDetails SharedMailbox
# Iterate through each shared mailbox
foreach ($mailbox in $sharedMailboxes) {
# Check if the user has full access to the shared mailbox
$fullAccessPermissions = Get-MailboxPermission $mailbox.Identity | Where-Object { $_.AccessRights -match "FullAccess" -and $_.User -like "*$userEmailAddress*" }
# If user has full access, remove the permission
if ($fullAccessPermissions) {
Remove-MailboxPermission -Identity $mailbox.Identity -User $userEmailAddress -AccessRights FullAccess -Confirm:$false
Write-Host "Full access permission removed for user $userEmailAddress from mailbox $($mailbox.DisplayName)."
}
}
The above PowerShell script will remove Full Access permission for [email protected] from all the shared mailboxes as shown below:

List all the users who have Full Access permission on shared mailboxes
In this scenario we will list all the users who have Full Access permission on shared mailboxes and will export the output to a CSV file.
Replace “C:\CSV Files\SharedMailboxPermissions.csv” with actual patch where you want to save the CSV file and run below PowerShell script:
# Get all shared mailboxes
$sharedMailboxes = Get-Mailbox -RecipientTypeDetails SharedMailbox
# Initialize an array to store results
$results = @()
# Iterate through each shared mailbox
foreach ($mailbox in $sharedMailboxes) {
# Get full access permissions for the shared mailbox excluding NT AUTHORITY\SELF
$fullAccessPermissions = Get-MailboxPermission $mailbox.Identity | Where-Object { $_.AccessRights -match "FullAccess" -and $_.User -ne "NT AUTHORITY\SELF" }
# Iterate through each permission
foreach ($permission in $fullAccessPermissions) {
$displayName = (Get-Mailbox $permission.User).DisplayName
$userEmailAddress = (Get-Mailbox $permission.User).PrimarySmtpAddress
# Add the result to the results array
$results += [PSCustomObject]@{
SharedMailboxDisplayName = $mailbox.DisplayName
SharedMailboxEmailAddress = $mailbox.PrimarySmtpAddress
UserDisplayName = $displayName
UserEmailAddress = $userEmailAddress
}
}
}
# Export the results to a CSV file
$results | Export-Csv -Path "C:\CSV Files\SharedMailboxPermissions.csv" -NoTypeInformation
The above script will fetch the users who have Full Access permission on shared mailboxes and will export the results in a CSV file as shown below:
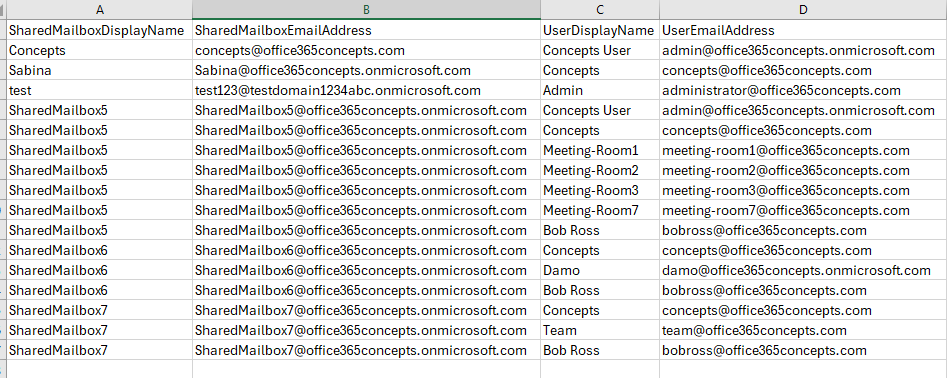
Remove bulk users access from shared mailboxes using CSV file
In this scenario we will use the same CSV file that we exported in the previous example, and will remove access for all the users from all shared mailboxes.
You can modify the CSV file as per your required and then you can use below PowerShell script.
We will modify the CSV file and will use 2 columns with name SharedMailboxEmailAddress and UserEmailAddress as shown below:
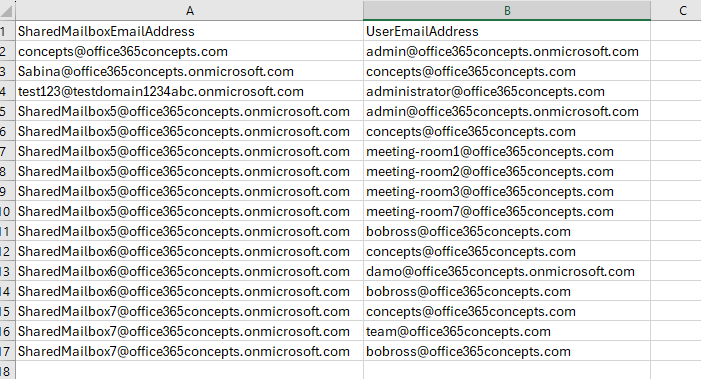
Replace “C:\CSV Files\SharedMailboxPermissions.csv” with the actual path of your CSV file and run below PowerShell script:
# Specify the path to the CSV file
$csvFilePath = "C:\CSV Files\SharedMailboxPermissions.csv"
# Import data from CSV file
$csvData = Import-Csv -Path $csvFilePath
# Iterate through each row in the CSV file
foreach ($row in $csvData) {
# Extract user and shared mailbox email addresses from the current row
$userEmailAddress = $row.UserEmailAddress
$sharedMailboxEmailAddress = $row.SharedMailboxEmailAddress
# Check if the user and shared mailbox email addresses are provided
if (-not [string]::IsNullOrWhiteSpace($userEmailAddress) -and -not [string]::IsNullOrWhiteSpace($sharedMailboxEmailAddress)) {
# Remove full access permission for the user from the shared mailbox
Remove-MailboxPermission -Identity $sharedMailboxEmailAddress -User $userEmailAddress -AccessRights FullAccess -Confirm:$false
Write-Host "Full access permission removed for user $userEmailAddress from shared mailbox $sharedMailboxEmailAddress."
} else {
Write-Host "Email addresses not provided for both user and shared mailbox in the CSV row."
}
}
Conclusion
In this blog you learnt how to list shared mailboxes on which a user has Full Access permission, how to remove user access from shared mailbox, how to list all the users who have Full Access permission on shared mailboxes, and how to remove bulk users access from shared mailboxes using CSV file.
You might like our other articles on Manage room mailboxes using PowerShell, Manage room mailbox permissions using PowerShell and Manage user mailboxes using PowerShell.
Please join us on YouTube for the latest videos on the Cloud technology and join our Newsletter for the early access of the blogs and updates.
Happy Scripting!!