Manage office 365 email forwarding using PowerShell
In this article you will learn how to manage Office 365 email forwarding using PowerShell.
Table of Contents
What is email forwarding in Office 365
Email forwarding feature allows users to automatically forward emails they receive in their Exchange Online mailbox to another email address. There are multiple ways in Exchange Online to forward emails to other users, but in this article we will talk about mailbox forwarding and how to manage mailbox forwarding using PowerShell.
As an administrator when you manage mailbox forwarding using PowerShell, you need to consider few things as discussed below:
With the help of Exchange Online mailbox forwarding, users can forward their emails to internal or external (outside the organization) mailboxes. To manage mailbox forwarding in Office 365, we use Get-Mailbox and Set-Mailbox PowerShell commands along with below parameters:
ForwardingAddress: The ForwardingAddress parameter specifies a forwarding email address of internal user (inside organization) to whom the emails are forwarded.
ForwardingSmtpAddress: The ForwardingSmtpAddress parameter specifies the email address of an external user (outside organization) to whom the emails are forwarded.
Along with above 2 parameters, we use DeliverToMailboxAndForward parameters to manage if the copy of the email should be saved in the mailbox before it is forwarded.
DeliverToMailboxAndForward is $true: Messages are saved to the mailbox and then forwarded to the specified recipient.
DeliverToMailboxAndForward is $false: Messages are only forwarded to the specified recipient. Messages aren’t saved to the mailbox.
Manage Office 365 email forwarding using PowerShell
Connect to Exchange Online PowerShell
Before you run below PowerShell commands, you need to connect to Exchange Online PowerShell. Open Windows PowerShell and run below command:
Connect-ExchangeOnline
Display information about email forwarding in Office 365
First let’s learn how to display the information about the email forwarding set on the Exchange Online mailboxes.
Display internal email forwarding for a mailbox
To display email forwarding set on a mailbox to forward emails to internal users, run below PowerShell command:
Get-Mailbox -Identity "[email protected]" | Select-Object ForwardingAddress
Display internal email forwarding for a mailbox and check if save copy is enabled
To display internal email forwarding for a mailbox and check if save a copy feature is enabled or not, run below PowerShell command:
Get-Mailbox -Identity "[email protected]" | Select-Object ForwardingAddress,DeliverToMailboxAndForward
The above command will display if user has enabled email forwarding to internal user, as well as if save a copy is enabled or not.

Display external email forwarding for a mailbox
If you want to verify if external email forwarding is set on a mailbox, run below PowerShell command:
Get-Mailbox -Identity "[email protected]" | Select-Object ForwardingSmtpAddress
Display external email forwarding for a mailbox and check if save copy is enabled
To display external email forwarding for a mailbox and check if save a copy feature is enabled or not, run below PowerShell command:
Get-Mailbox -Identity "[email protected]" | Select-Object ForwardingSmtpAddress,DeliverToMailboxAndForward
Display internal and external email forwarding for a mailbox
If you want to find the internal and external email forwarding for a mailbox, run below PowerShell command:
Get-Mailbox -Identity "[email protected]" | Select-Object ForwardingAddress,ForwardingSmtpAddress
Display internal and external email forwarding for a mailbox and check if save copy is enabled
If you want to find if a mailbox is forwarding his emails to both internal and external users and you want to verify if save a copy feature is enabled or not, run below PowerShell command:
Get-Mailbox "[email protected]" | Select-Object ForwardingAddress,ForwardingSmtpAddress,DeliverToMailboxAndForward
The above command will display all 3 parameters (ForwardingAddress, ForwardingSmtpAddress, DeliverToMailboxAndForward) for a mailbox as shown below:

Display internal email forwarding of all users
To display internal email forwarding for all the users, run below PowerShell command:
Get-Mailbox -ResultSize Unlimited | Select-Object DisplayName,ForwardingAddress
Display external email forwarding of all users
To display external email forwarding for all the users, run below PowerShell command:
Get-Mailbox -ResultSize Unlimited | Select-Object DisplayName,ForwardingSmtpAddress
Display internal and external email forwarding of all users
To display internal and external email forwarding for all the users, run below PowerShell command:
Get-Mailbox -ResultSize Unlimited | Select-Object DisplayName,ForwardingAddress,ForwardingSmtpAddress
Display internal and external email forwarding information of all users and verify save copy feature
To display internal and external email forwarding for all the users, and verify if save a copy feature is enabled or not, run below PowerShell command:
Get-Mailbox -ResultSize Unlimited | Select-Object DisplayName,ForwardingAddress,ForwardingSmtpAddress,DeliverToMailboxAndForward
Export email forwarding information of users to CSV who have email forwarding enabled
If your requirement is to find all the users on which email forwarding is enabled, run below PowerShell script:
# Get all mailboxes with forwarding enabled
$mailboxes = Get-Mailbox -Filter {ForwardingSmtpAddress -ne $null -or ForwardingAddress -ne $null}
# Initialize an array to store mailbox forwarding information
$mailboxForwardingInfo = @()
# Loop through each mailbox
foreach ($mailbox in $mailboxes) {
$mailboxInfo = [PSCustomObject]@{
DisplayName = $mailbox.DisplayName
EmailAddress = $mailbox.PrimarySmtpAddress
ForwardingAddress = $null
ForwardingSmtpAddress = $null
}
# Check if forwarding is set to an external address
if ($mailbox.ForwardingSmtpAddress -ne $null) {
$mailboxInfo.ForwardingSmtpAddress = $mailbox.ForwardingSmtpAddress
}
# Check if forwarding is set to an internal address
if ($mailbox.ForwardingAddress -ne $null) {
$mailboxInfo.ForwardingAddress = $mailbox.ForwardingAddress
}
# Add mailbox forwarding information to the array
$mailboxForwardingInfo += $mailboxInfo
}
# Export the mailbox forwarding information to a CSV file
$mailboxForwardingInfo | Export-Csv -Path "C:\CSV Files\MailboxForwardingInfo.csv" -NoTypeInformation
The above script retrieves all mailboxes with forwarding enabled. It iterates through each mailbox and checks if forwarding is set to an internal or external address. Then it stores the mailbox forwarding information in a custom object array and exports the mailbox forwarding information to a CSV file named “MailboxForwardingInfo.csv” as shown below:

Set up email forwarding in Office 365
Set up internal email forwarding for a user without save copy
To set up internal email forwarding for a user, use below PowerShell command:
Set-Mailbox -Identity "[email protected]" -ForwardingAddress "[email protected]"
Set up external email forwarding for a user with save copy
To set up external email forwarding for a user, use below PowerShell command:
Set-Mailbox -Identity "[email protected]" -ForwardingSmtpAddress "[email protected]" -DeliverToMailboxAndForward $true
Set up internal and external email forwarding for a user without save copy
To set up internal and external email forwarding for a user without saving a copy of the email, use below PowerShell command:
Set-Mailbox -Identity "[email protected]" -ForwardingAddress "[email protected]" -ForwardingSmtpAddress "[email protected]"
Set up internal and external email forwarding for a user with save copy enabled
To set up internal and external email forwarding for a user with save copy feature enabled, use below PowerShell command:
Set-Mailbox -Identity "[email protected]" -ForwardingAddress "[email protected]" -ForwardingSmtpAddress "[email protected]" -DeliverToMailboxAndForward $true
Set up email forwarding using CSV file
If your requirement is to set email forwarding using a CSV file, follow below steps:
Create CSV file: Create a CSV file with below columns:
Identity: Email address of the mailbox for which you want to enable email forwarding.
ForwardingAddress: Email address of internal user to whom emails should be forwarded.
ForwardingSmtpAddress: Email address of the external user to whom emails should be forwarded.
DeliverToMailboxAndForward: Whether a copy of the email needs to be saved before email is forwarded to the recipient.

Modify the path of CSV file and run below PowerShell script.
# Define the path to the CSV file
$csvPath = "C:\CSV Files\forwarding.csv"
# Read the CSV file
$forwardingSettings = Import-Csv -Path $csvPath
# Loop through each row in the CSV file
foreach ($setting in $forwardingSettings) {
$identity = $setting.Identity
$forwardingAddress = $setting.ForwardingAddress
$forwardingSmtpAddress = $setting.ForwardingSmtpAddress
$deliverToMailboxAndForward = [System.Convert]::ToBoolean($setting.DeliverToMailboxAndForward)
# Check if forwarding address is empty
if ([string]::IsNullOrWhiteSpace($forwardingAddress)) {
Write-Host "Forwarding address is empty for $identity. Skipping..."
continue
}
# Set up forwarding for the mailbox
Set-Mailbox -Identity $identity -ForwardingAddress $forwardingAddress -ForwardingSmtpAddress $forwardingSmtpAddress -DeliverToMailboxAndForward $deliverToMailboxAndForward
}
Set up email forwarding using Custom Attribute
In this scenario, we will set up email forwarding on the mailboxes whose CustomAttribute1 is set to Test.
The script that we are going to use in this scenario, will set up email forwarding on the mailboxes on which CustomAttribute1 is set to Test, it will enable ForwardingAddress and ForwardingSmtpAddress, and will enable DeliverToMailboxAndForward parameter. Administrator will get a prompt to enter the ForwardingAddress and ForwardingSmtpAddress during running this script.
# Get forwarding address and SMTP address from user input
$forwardingAddress = Read-Host "Enter the forwarding address:"
$forwardingSmtpAddress = Read-Host "Enter the forwarding SMTP address (if different from forwarding address):"
# Get mailboxes with CustomAttribute1 set to "Test"
$mailboxes = Get-Mailbox -Filter {CustomAttribute1 -eq "Test"}
# Initialize an array to store email addresses of users with forwarding enabled
$forwardingUsers = @()
# Loop through each mailbox
foreach ($mailbox in $mailboxes) {
# Set up forwarding for the mailbox
Set-Mailbox -Identity $mailbox.Identity -ForwardingAddress $forwardingAddress -ForwardingSmtpAddress $forwardingSmtpAddress -DeliverToMailboxAndForward $true
# Add the email address to the array
$forwardingUsers += $mailbox.PrimarySmtpAddress
}
# Display the email addresses of users with forwarding enabled
Write-Host "Forwarding is set up for the following users:"
$forwardingUsers
The above script will also display the email addresses of the users for whom email forwarding is enabled as shown below:

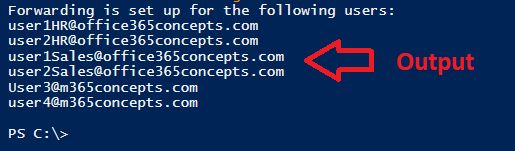
Set up email forwarding using Department attribute
In this scenario we will set up email forwarding on the mailboxes whose Department attribute is set to IT.
# Get forwarding address and SMTP address from user input
$forwardingAddress = Read-Host "Enter the forwarding address:"
$forwardingSmtpAddress = Read-Host "Enter the forwarding SMTP address (if different from forwarding address):"
# Get mailboxes and filter based on Department attribute
$mailboxes = Get-Recipient -RecipientTypeDetails UserMailbox | Where-Object { $_.CustomAttribute1 -eq "IT" }
# Initialize an array to store email addresses of users with forwarding enabled
$forwardingUsers = @()
# Check if any mailboxes are found in the IT department
if ($mailboxes.Count -eq 0) {
Write-Host "No mailboxes found in the IT department."
} else {
# Loop through each mailbox
foreach ($mailbox in $mailboxes) {
# Set up forwarding for the mailbox
try {
Set-Mailbox -Identity $mailbox.Identity -ForwardingAddress $forwardingAddress -ForwardingSmtpAddress $forwardingSmtpAddress -DeliverToMailboxAndForward $true -ErrorAction Stop
# Add the email address to the array
$forwardingUsers += $mailbox.PrimarySmtpAddress
} catch {
Write-Host "Error setting up forwarding for $($mailbox.PrimarySmtpAddress): $_"
}
}
# Display the email addresses of users with forwarding enabled
if ($forwardingUsers.Count -gt 0) {
Write-Host "Forwarding is set up for the following users in the IT department:"
$forwardingUsers
}
}
The above script will find the users who have Department attribute set to IT, and enables email forwarding. Administrator will receive the prompt to add ForwardingAddress and ForwardingSmtpAddress while running the script.
Disable email forwarding Office 365
Disable email forwarding for a user
To disable internal email forwarding for a user, use below PowerShell command:
Set-Mailbox -Identity "[email protected]" -ForwardingAddress $null -ForwardingSmtpAddress $null -DeliverToMailboxAndForward $false
The above command will disable internal and external email forwarding for [email protected] and will disable save copy feature.
Disable email forwarding using CSV file
In this scenario we will use a CSV file as shown below to disable email forwarding for specific users:
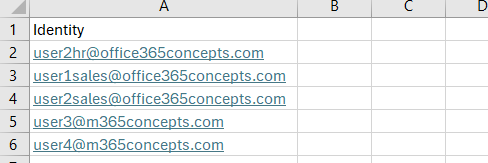
# Define the path to the CSV file
$csvPath = "C:\CSV FIles\testing.csv"
# Read the CSV file
$identities = Import-Csv -Path $csvPath
# Loop through each row in the CSV file
foreach ($identity in $identities) {
$user = $identity.Identity
# Check if forwarding is already disabled for the mailbox
$mailbox = Get-Mailbox -Identity $user
if (-not $mailbox.ForwardingSmtpAddress -and -not $mailbox.ForwardingAddress -and -not $mailbox.DeliverToMailboxAndForward) {
Write-Host "Forwarding was already disabled for the mailbox $user."
continue
}
# Disable forwarding for the mailbox
try {
Set-Mailbox -Identity $user -ForwardingSmtpAddress $null -ForwardingAddress $null -DeliverToMailboxAndForward $false -ErrorAction Stop
Write-Host "Email forwarding disabled for $user."
} catch {
$errorMessage = $_.Exception.Message
Write-Host "Error disabling email forwarding for $($user): $errorMessage"
}
}
The above PowerShell script will disable the email forwarding for the users mentioned within the CSV file. If email forwarding was not enabled for any mailbox, script will display “Forwarding was already disabled for the mailbox” as shown below:

Disable email forwarding using Custom Attribute
In this scenario we will disable email forwarding for the users whose CustomAttribute1 is set to Test.
# Get all mailboxes and filter those with CustomAttribute1 set to "Test"
$mailboxes = Get-Mailbox -ResultSize Unlimited | Where-Object { $_.CustomAttribute1 -eq "Test" }
# Loop through each mailbox
foreach ($mailbox in $mailboxes) {
$user = $mailbox.Identity
# Check if forwarding is already disabled for the mailbox
if (-not $mailbox.ForwardingSmtpAddress -and -not $mailbox.ForwardingAddress -and -not $mailbox.DeliverToMailboxAndForward) {
Write-Host "Forwarding was already disabled for the mailbox $user."
continue
}
# Disable forwarding for the mailbox
try {
Set-Mailbox -Identity $user -ForwardingSmtpAddress $null -ForwardingAddress $null -DeliverToMailboxAndForward $false -ErrorAction Stop
Write-Host "Email forwarding disabled for $user."
} catch {
$errorMessage = $_.Exception.Message
Write-Host "Error disabling email forwarding for $($user): $errorMessage"
}
}
The above script will find he users who have CustomAttribute1 set to Test, then script will disable email forwarding for those users and display the output. This will also display if email forwarding was not enabled already for any user as shown below:

Conclusion
In this blog you learnt how to manage Office 365 email forwarding using PowerShell. You learnt how to export email forwarding information of users to CSV file, how to set up email forwarding in Office 365, how to disable email forwarding Office 365, and how to set up email forwarding using CSV file. You might like our other articles on Manage Office 365 Licenses using PowerShell, Manage Dynamic Distribution Groups using PowerShell and Manage Distribution Groups using PowerShell.
If you found this article helpful and informative, please share it within your community and do not forget to share your feedback in the comments below.
Please join us on our YouTube channel for the latest videos on Cloud technology, and join our Newsletter for the early access of the articles and updates.
Happy Scripting!!