Manage users in Office 365 using PowerShell
In this article you will learn how to manage users in Office 365 using PowerShell. You will learn how to create users in Office 365 using PowerShell, how to delete users, and how to restore users in Office 365 using PowerShell commands.
Table of Contents
Manage users in Office 365 using PowerShell
With PowerShell, you can streamline user management tasks such as creating, updating, and removing user accounts, assigning licenses, resetting passwords, and managing group memberships. Gain control over your Office 365 user environment with the flexibility and automation capabilities of PowerShell, simplifying administrative tasks and ensuring smooth user management workflows.
Create user accounts in Office 365 using PowerShell
Connect to MSOnline PowerShell
Before you run below PowerShell commands, you need to connect to MSOnline PowerShell. Open Windows PowerShell as Administrator, run below PowerShell command and type global administrator credentials when you are prompted:
Install-Module MSOnline
Import-Module MSOnline
Connect-MsolService
Create a user using PowerShell
In this example we will create a user account with name “Bob Ross” using PowerShell command:
New-MsolUser -UserPrincipalName "[email protected]" -DisplayName "Bob Ross" -FirstName "Bob" -LastName "Ross"
The above PowerShell command will create a new user account with Display Name “Bob Ross” and UserPrincipalName “[email protected]”, and it will generate a random password for the user account as shown below:

Create a user and set password using PowerShell
In this example we will create a user account and we will set a password for this account using PowerShell command.
To set password for the user account, we will use Password switch along with New-MSolUser command as shown below:
New-MsolUser -UserPrincipalName "[email protected]" -DisplayName "John Smith" -FirstName "John" -LastName "Smith" -Password "John@1234"
The above command will create a new user account with UserPrincipalName as “[email protected]” and it will set a password “John@1234” for this account. When the user will login for first time, he will receive prompt to set a new password.
Create a user and set password never expire using PowerShell
In this example we will create a new user account, we will assign a new password, and we will set the password to never expire.
To achieve this we will use below parameters along with New-MSolUser PowerShell command:
- Password: We will use Password parameter to set a new password for the user. The user will be prompted to set a new password on the first login attempt.
- PasswordNeverExpires: This parameter will set the password to never expire. That means the password for user account will never expire.
Run below PowerShell command and make sure to update it as per your requirement:
New-MsolUser -UserPrincipalName "[email protected]" -DisplayName "Bob Ross" -FirstName "Bob" -LastName "Ross" -Password "Match@1234" -PasswordNeverExpires $true
Create a user and enable MFA using PowerShell
In this example we will create a new user and we will enable Multi Factor Authentication using PowerShell.
To achieve our requirement, we will use StrongAuthenticationRequirement parameters with Set-MSolUser command.
In the below script, replace “[email protected]“, “Bob Ross“, “Bob“, “Ross“, “IN“, and “P@ssw0rd” with the actual values you want to use for the new user, and run it.
# Define user details
$userDetails = @{
UserPrincipalName = "[email protected]"
DisplayName = "Bob Ross"
FirstName = "Bob"
LastName = "Ross"
UsageLocation = "IN" # Specify the usage location (e.g., IN)
Password = "P@ssw0rd" # Specify the initial password
}
# Create the new user
New-MsolUser @userDetails
# Enable Multi-Factor Authentication for the user
$userPrincipalName = $userDetails["UserPrincipalName"]
# Get the user object
$user = Get-MsolUser -UserPrincipalName $userPrincipalName
# Define MFA settings
$strongAuth = New-Object -TypeName Microsoft.Online.Administration.StrongAuthenticationRequirement
$strongAuth.RelyingParty = "*"
$strongAuth.State = "Enabled"
# Apply MFA settings to the user
Set-MsolUser -UserPrincipalName $userPrincipalName -StrongAuthenticationRequirements @($strongAuth)
The above script will create a new user and then enables Multi-Factor Authentication (MFA) for that user by setting the StrongAuthenticationRequirements property.
Create bulk users in Office 365 using PowerShell and CSV
In this example we will create bulk users in Office 365 using PowerShell and CSV file.
First we will create a CSV file with below details:
UserPrincipalName: This column will contain the user name for the users.
DisplayName: This column will contain the display name for the users.
FirstName: This column will contain the first name of the users.
LastName: This column will contain the last name of the users.
Password: This column will contain password for each user.
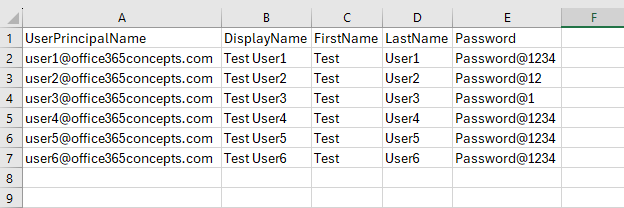
Modify the CSV file path and run below PowerShell script.
# Import CSV file
$UserList = Import-Csv -Path "C:\csv files\CreateBulkUser.csv"
# Loop through each user in the CSV file
foreach ($User in $UserList) {
$UserPrincipalName = $User.UserPrincipalName
$DisplayName = $User.DisplayName
$FirstName = $User.FirstName
$LastName = $User.LastName
$Password = $User.Password
# Create the user
$NewUser = New-MsolUser -UserPrincipalName $UserPrincipalName -DisplayName $DisplayName -FirstName $FirstName -LastName $LastName -Password $Password
Write-Host "User created: $($NewUser.UserPrincipalName)"
}
The above PowerShell script will create users and update their account properties as specified within CSV file, and will display the output as shown below:
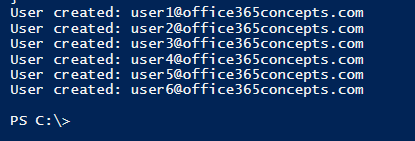
Manage user account properties using PowerShell
Disable sign in for a user using PowerShell
In this example we will block sign in of a user using PowerShell command. When we disable or block sign in of a user, that user will not be able to login to Microsoft 365 or Azure applications.
To disable sign in of a user, we will use Set-MSolUser command along with BlockCredential parameter.
Set-MsolUser -UserPrincipalName "[email protected]" -BlockCredential $True
The above command will block User1 to be able to sign in to Microsoft 365 or Azure applications.
Bulk disable sign in for users using PowerShell and CSV
In this example we will use PowerShell script and CSV file to block sign in for multiple users in Office 365 environment.
We will create a CSV file with column UserName that will contain UserPrincipalName for each user as shown below:
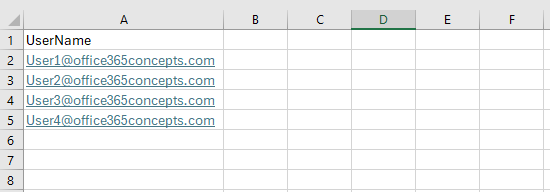
Next, we will use a PowerShell script to block sign in for users using the above CSV file. Please ensure to modify the path of CSV file and run below PowerShell script.
# Define the path to the CSV file containing user information
$csvFilePath = "C:\csv files\blocksignin.csv"
# Import the CSV file
$userList = Import-Csv -Path $csvFilePath
# Loop through each user in the CSV file and disable sign-in
foreach ($user in $userList) {
$userName = $user.UserName
Set-MsolUser -UserPrincipalName $userName -BlockCredential $true
Write-Host "Disabled sign-in for user: $userName"
}
Write-Host "Sign-in disabled for all users listed in the CSV file."
The above PowerShell script will iterate through each user listed in the CSV file, disable sign-in for each user in Office 365, and display a message indicating that sign-in has been disabled for each user. Finally, it will display a confirmation message when the process is complete as shown below:

Disable sign in for users using Department attribute
In this example we will block sign in for users in Office 365 whose Department attribute is set to “HR”.
We have 4 users whose Department attribute is set to “HR” as shown below:
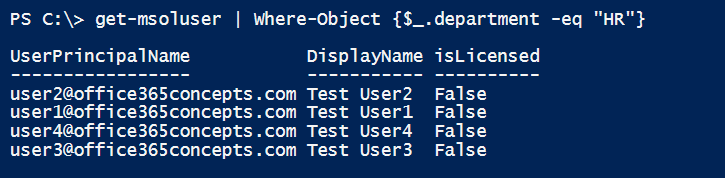
We will use below PowerShell script to block sign in for these 4 users.
# Get users with department attribute set to "HR"
$hrUsers = Get-MsolUser -All | Where-Object { $_.Department -eq "HR" }
# Disable sign-in for HR users
foreach ($user in $hrUsers) {
Set-MsolUser -UserPrincipalName $user.UserPrincipalName -BlockCredential $true
Write-Host "Disabled sign-in for user $($user.UserPrincipalName) in HR department."
}
Write-Host "Sign-in disabled for all users in the HR department."
The above PowerShell script will retrieve all users with the Department attribute set to “HR”, it will disable sign-in for each user found in the HR department, and will display the message for each user whose sign-in has been disabled. And finally, it will display a confirmation message as shown below:

Set password never expires for a user using PowerShell
In this example, we will set a user’s password to never expire so that the user is never asked to change his password.
To achieve this we will use Set-MSolUser PowerShell command along with PasswordNeverExpires parameter as shown below:
Set-MsolUser -UserPrincipalName "[email protected]" -PasswordNeverExpires $true
The above command will set User1’s account password to never expire so that User1 is never asked to change his password.
Bulk set password never expires using PowerShell and CSV
In this example, we will use PowerShell script and CSV file to set password never expires for multiple users.
We will create a CSV file with below columns:
- UserName: This column will include the UserPrincipalName for the each user.
- PasswordExpires: This column will include the value Never.
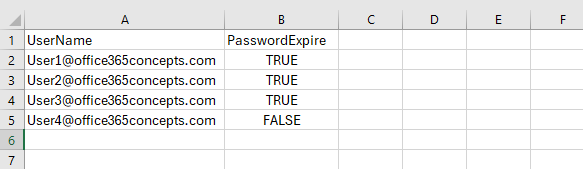
Replace “C:\csv files\passwordneverexpire.csv” with the actual path to your CSV file, and run below PowerShell sccript:
# Path to the CSV file
$csvPath = "C:\csv files\passwordneverexpire.csv"
# Read the CSV file
$userData = Import-Csv $csvPath
# Loop through each row in the CSV file
foreach ($user in $userData) {
$username = $user.username
$passwordExpire = $user.passwordexpire.Trim().ToLower()
# Check if the username exists
$userDetails = Get-MsolUser -UserPrincipalName $username -ErrorAction SilentlyContinue
if ($userDetails -ne $null) {
# Set password to never expire if passwordexpire is 'true'
if ($passwordExpire -eq "true") {
Set-MsolUser -UserPrincipalName $username -PasswordNeverExpires $true
Write-Host "Password for user $username set to never expire."
} else {
Write-Host "Password expiration for user $username left as default."
}
} else {
Write-Host "User $username not found."
}
}
The above PowerShell script reads a CSV file with two columns (UserName and PasswordExpire) and sets the password expiration settings for each user in Office 365. If the PasswordExpire column contains “True“, it sets the password to never expire for that user. Otherwise, it leaves the password expiration setting as default as shown below:

Enable MFA for a user using PowerShell
In this example we will enable MFA (Multi Factor Authentication) for a user.
# Set the user's UPN (User Principal Name)
$userUPN = "[email protected]" # Replace with the UPN of the user
# Retrieve the user object
$user = Get-MsolUser -UserPrincipalName $userUPN
if ($user) {
# Create StrongAuthenticationRequirement object
$strongAuth = New-Object -TypeName Microsoft.Online.Administration.StrongAuthenticationRequirement
$strongAuth.RelyingParty = "*"
$strongAuth.State = "Enabled"
# Enable MFA for the user
Set-MsolUser -UserPrincipalName $userUPN -StrongAuthenticationRequirements $strongAuth
Write-Host "MFA enabled successfully for user $userUPN."
} else {
Write-Host "User $userUPN not found."
}
Enable MFA for all users in Office 365 using PowerShell
In this example we will enable MFA for all users in Office 365 using PowerShell script.
# Get all users in the tenant
$users = Get-MsolUser -All
# Iterate through each user and enable MFA
foreach ($user in $users) {
$userUPN = $user.UserPrincipalName
# Create StrongAuthenticationRequirement object
$strongAuth = New-Object -TypeName Microsoft.Online.Administration.StrongAuthenticationRequirement
$strongAuth.RelyingParty = "*"
$strongAuth.State = "Enabled"
# Enable MFA for the user
Set-MsolUser -UserPrincipalName $userUPN -StrongAuthenticationRequirements $strongAuth
Write-Host "MFA enabled successfully for user $userUPN."
}
The above script will retrieve all users in the Office 365 tenant using Get-MsolUser -All. For each user, the script will create a StrongAuthenticationRequirement object, set its properties to enable MFA, and then use Set-MsolUser to enable MFA for that user. Finally, it will display a message indicating that MFA was enabled successfully for each user.
Convert user accounts
In Azure Active Directory (Microsoft Entra ID), there are different types of user accounts, including Member users and Guest users.
- Member users are typically internal users within your organization. These users are directly associated with your Azure AD tenant. Member users can access resources and services within your organization’s Azure AD tenant based on the permissions and roles assigned to them.
- Guest users are external users who are invited to collaborate with your organization. These users are invited to access specific resources or applications within your organization’s Azure AD tenant. Guest users have limited access compared to member users. They can access only the resources and applications they are invited to, and their access is controlled by Azure AD B2B (Business-to-Business) collaboration settings.
Convert member user to guest user
In this example, we will convert a member user (regular user) to guest user.
Set-MsolUser -UserPrincipalName "[email protected]" -UserType Guest
To verify if the user account is converted to guest user, run below PowerShell command:
Get-MsolUser -UserPrincipalName "[email protected]" | Select-Object UserType
Convert guest user to member user
In this example we will convert a guest user account to member user:
Set-MsolUser -UserPrincipalName "[email protected]" -UserType Member
To verify if the account is converted to member user, run below PowerShell command:
Get-MsolUser -UserPrincipalName [email protected] | Select-Object UserType
Delete user accounts in Office 365 using PowerShell
Now we will learn how to delete and restore user accounts using PowerShell commands.
Soft Delete vs Hard Delete Office 365
Soft delete a user account: In Office 365, when we delete a user account using PowerShell or from Microsoft 365 Admin Center or from Microsoft Entra ID, that user account goes to Deleted Users (recycle bin) section, where it is retained for 30 days. An administrator can use PowerShell to restore the user account or can do it from Microsoft Entra ID portal. But if the user account is not restored within 30 days, the account will be permanently purged and can not be recovered. To soft delete a user in Office 365, we use Remove-MSolUser command, and to verify the users within Deleted Users section, we use Get-MsolUser command along with ReturnDeletedUsers parameter.
Hard delete a user account: As explained above, when we delete a user account, it is retained in Deleted Users section for 30 days. If an administrator deletes this account from Deleted Users section as well, the account is deleted permanently and can not be recovered. To hard delete a user in Office 365 we use Remove-MSolUser command along with RemoveFromRecycleBin parameter.
Soft delete a user in Office 365
To soft delete a user in Office 365, we will use Remove-MSolUser PowerShell command as described below:
Remove-MsolUser -UserPrincipalName "[email protected]" -Force
The above PowerShell command will soft delete the user and will send it to Deleted Users section. -Force will not display a prompt to confirm if you want to delete this user or not.
To verify users in Deleted Users (recycle bin) section, run below PowerShell command:
Get-MsolUser -ReturnDeletedUsers
The above PowerShell command will list all the accounts those are retained within Deleted Items section as shown below:
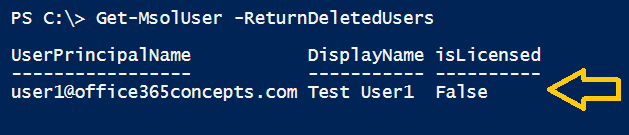
Bulk soft delete users using PowerShell and CSV
In this example, we will soft delete multiple users using PowerShell and CSV file.
We will create a CSV file with a column UserPrincipalName which will contain UPN of each user as shown below:
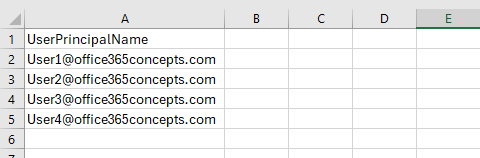
Replace “C:\csv files\BulkDeleteUsers.csv” with the actual path to your CSV file and run below PowerShell script:
# Path to the CSV file containing user principal names (replace with your CSV path)
$csvPath = "C:\csv files\BulkDeleteUsers.csv"
# Import CSV file
$userPrincipalNames = Import-Csv -Path $csvPath | Select-Object -ExpandProperty UserPrincipalName
# Iterate over each user principal name in the CSV
foreach ($userPrincipalName in $userPrincipalNames) {
# Soft delete the user
Write-Host "Soft deleting user: $userPrincipalName"
Remove-MsolUser -UserPrincipalName $userPrincipalName -Force
}
Write-Host "Soft deletion of users completed."
The above PowerShell script will read the CSV file containing the UserPrincipalName column, then iterate over each UserPrincipalName and soft delete the corresponding user in Office 365.
Restore a user in Office 365
In this example we will restore a user using PowerShell command. To restore a user in Office 365, we will use Restore-MsolUser command as shown below:
Restore-MsolUser -UserPrincipalName "[email protected]"
If the above PowerShell command fails to restore the user account due t proxy address conflicts, use AutoReconcileProxyConflicts parameter as shown below:
Restore-MsolUser -UserPrincipalName "[email protected]" -AutoReconcileProxyConflicts
The AutoReconcileProxyConflicts parameter removes the conflicting proxy addresses while restoring the user accounts.
Bulk restore users in Office 365 using PowerShell
In this example we will restore all the user accounts in Office 365 using PowerShell.
To restore all the user accounts, first we will collect all the user identities from Deleted Users container using Get-MSolUser PowerShell command along with ReturnDeletedUsers parameter, and then we will use Restore-MsolUser command along with AutoReconcileProxyConflicts parameter as shown below:
Get-MsolUser -ReturnDeletedUsers | Restore-MsolUser -AutoReconcileProxyConflicts
Hard delete user in Office 365 using PowerShell
As we discussed above, when we hard delete user in Office 365, that user account is deleted permanently, and can not be recovered.
Note: You can not hard delete a user account directly using RemoveFromRecycleBin parameter. To hard delete a user in Office 365, you need to first soft delete a user, and then remove it permanently (hard delete).
To hard delete a user who is currently in Deleted Users container, run below PowerShell command:
Remove-MsolUser -UserPrincipalName "[email protected]" -RemoveFromRecycleBin -Force
Bulk hard delete users in Office 365 using PowerShell
To hard delete all users who are currently in Deleted Users container, run below PowerShell command:
Get-MsolUser -ReturnDeletedUsers | Remove-MsolUser -RemoveFromRecycleBin -Force
Hard delete users using Department attribute
In this scenario, we will hard delete users from Office 365 whose Department attribute is set to HR.
To achieve our requirement, first we will use Get-MsolUser command along with ReturnDeletedUsers parameter to collect all the users from Deleted Users container. Then we will use Select-Object switch to filter the users with Department attribute. And finally, we will use Remove-MsolUser command along with RemoveFromRecycleBin parameter to hard delete these users.
Get-MsolUser -ReturnDeletedUsers | Where-Object {$_.Department -eq "HR"} | Remove-MsolUser -RemoveFromRecycleBin -Force
Conclusion
In this article we learnt how to manage users in Office 365 using PowerShell, we learnt how to create bulk users in Office 365 using PowerShell, we talked about Soft delete vs Hard delete Office 365 concept, we learnt how to soft delete a user in Office 365, how to restore users in Office 365 using PowerShell, and how to hard delete user in Office 365 using PowerShell.
If you found this article helpful and informative, please share it within your community and share your feedback in the comments below.
You might like our other articles on Manage Office 365 licenses using PowerShell, Manage shared mailbox using PowerShell, Manage email forwarding using PowerShell, Manage Dynamic Distribution Groups (DDG) using PowerShell and Manage Distribution Groups (DG) using PowerShell.
Join us on YouTube for the latest videos on the Cloud technology and join our Newsletter for the early access of the articles and updates.
Happy Scripting!!