Manage Office 365 licenses with PowerShell
In this article you will learn how to manage Office 365 licenses with PowerShell.
Table of Contents
Watch the video
If you prefer to watch video to learn how to use PowerShell to manage specific tasks in Office 365, please watch this playlist.
How to Manage Office 365 licenses with PowerShell
Managing Office 365 licenses efficiently is crucial for organizations to optimize resource allocation and ensure users have access to the right services. PowerShell provides powerful automation capabilities to streamline license management tasks in Office 365 environments. With PowerShell, administrators can automate license assignment, track license usage, and troubleshoot issues effectively.
Connect to Microsoft Online PowerShell
Before your run below PowerShell commands or scripts, you need to connect to Microsoft Online (MSOnline). Run below command in Windows PowerShell:
Connect-MsolService
List all the licenses in Office 365 tenant
If you want to view all the licenses/subscriptions in your Office 365 tenant, run below PowerShell command:
Get-MsolAccountSku
The above command Get-MsolAccountSku will display all the subscriptions you have in Office 365 tenant along with the number of licenses you have, it will show how many licenses are assigned and the number of warning units.

If you want to display all the properties of the licenses (AccountName, AccountObjectId, AccountSkuId, ServiceStatus, SkuId, SuspendedUnits, and other properties), run below PowerShell command:
Get-MsolAccountSku | fl
The above command will display all the properties of the licenses that you have in your Office 365 tenant as shown below:
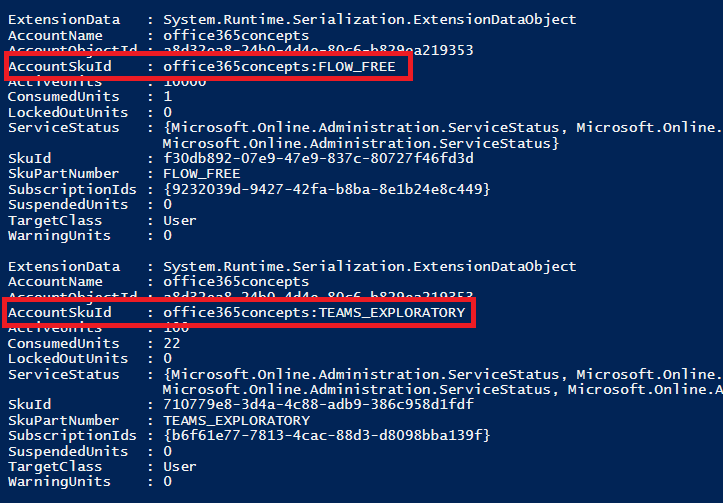
Check status of all the licenses in Office 365 tenant
If you want to check the status of all the licenses in Office 365 tenant, run below PowerShell command:
Get-MsolSubscription | fl
The above command will display all the properties of the licenses as shown below:
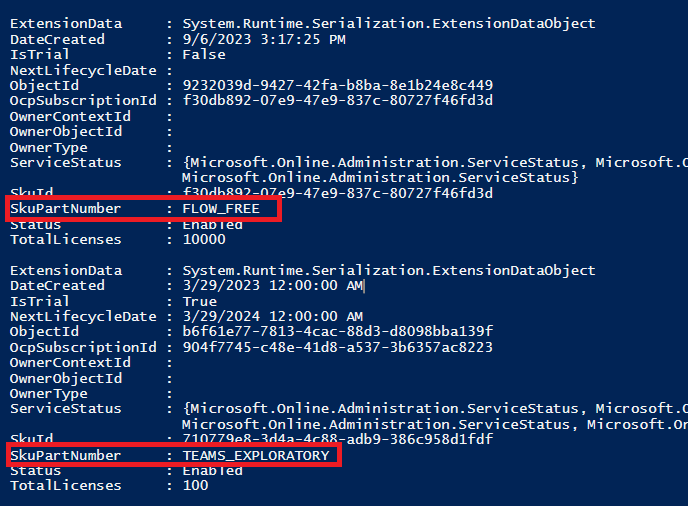
List services included in a license
If you want to list all the services included within a particular license, run below PowerShell command:
Get-MsolAccountSku | Select-Object AccountSkuId
The above command will list AccountSkuId of all the subscriptions of your tenant as shown below.
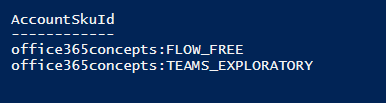
Copy the AccountSkuId for a particular license for which you want to see services, and replace “AccountSkuId Name” with this value:
Get-MsolAccountSku | Where-Object { $_.AccountSkuId -eq "AccountSkuId Name" } | Select-Object -ExpandProperty ServiceStatus
The exact command will look like below:
Get-MsolAccountSku | Where-Object { $_.AccountSkuId -eq "office365concepts:TEAMS_EXPLORATORY" } | Select-Object -ExpandProperty ServiceStatus
The above command will list all the services included in Teams Exploratory license as shown below:
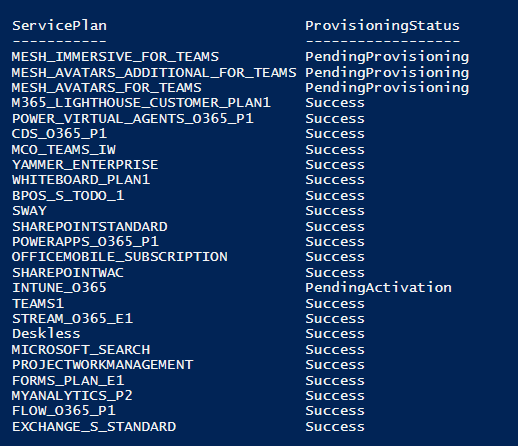
List services included in all licenses
If you want to list services included within all the licenses in your Office 365 Tenant, run below PowerShell script:
# Get services included in a specific license
Get-MsolAccountSku | ForEach-Object {
Write-Host "License $($PSItem.AccountSkuId) Services:"
$PSItem.ServiceStatus | ForEach-Object {
Write-Host "- $($_.ServicePlan.ServiceName)"
}
Write-Host "==============================="
}
The above script will list all the licenses of your tenant along with the services included within each license as shown below:
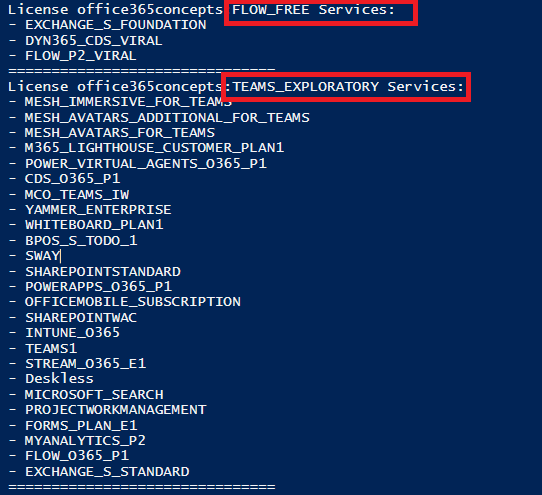
In the above image you can see I have 2 subscriptions in my Office 365 tenant (Flow Free and Teams Exploratory) and the script is displaying all the services included in each subscription.
Check expired licenses in Office 365 tenant
If you want to list all the expired licenses in your Office 365 tenant, run below PowerShell script:
# Get all account SKUs (licenses) in the tenant
$AccountSkus = Get-MsolAccountSku
# Get the current date
$CurrentDate = Get-Date
# Iterate through each account SKU
foreach ($AccountSku in $AccountSkus) {
$AccountSkuId = $AccountSku.AccountSkuId
Write-Host "License: $AccountSkuId"
# Get detailed information about the account SKU
$DetailedSkuInfo = Get-MsolAccountSku -AccountSkuId $AccountSkuId
# Display service plan details
foreach ($ServicePlan in $DetailedSkuInfo.ServiceStatus) {
$ServicePlanName = $ServicePlan.ServicePlan.ServiceName
$ProvisioningStatus = $ServicePlan.ProvisioningStatus
$EndDate = $ServicePlan.ServicePlanEndDate
# Check if the end date is before the current date (expired)
if ($EndDate -lt $CurrentDate) {
Write-Host "Service Plan: $ServicePlanName"
Write-Host "Provisioning Status: $ProvisioningStatus"
Write-Host "End Date: $EndDate (Expired)"
Write-Host "==============================="
}
}
}
The above script will list all the expired licenses as shown in the below image:
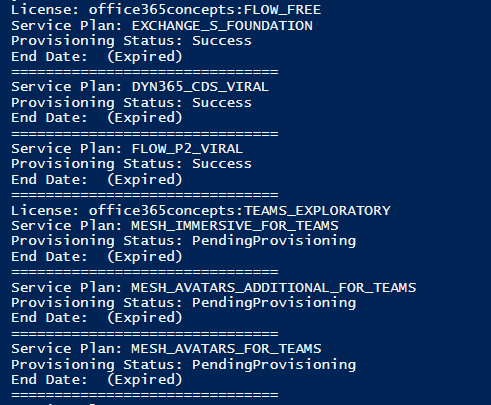
Find licenses expiration date
If you want to find the expiration date of licenses in Office 365, run below PowerShell command:
# Get all subscriptions (licenses) in the tenant
$Subscriptions = Get-MsolSubscription
# Iterate through each subscription
foreach ($Subscription in $Subscriptions) {
$SubscriptionName = $Subscription.SkuPartNumber
$NextLifeCycleDate = $Subscription.NextLifeCycleDate
Write-Host "Subscription: $SubscriptionName"
Write-Host "Next Life Cycle Date: $NextLifeCycleDate"
Write-Host "==============================="
}
The above script will list all the licenses along with their expiration date (Next Life Cycle Date) as shown below:
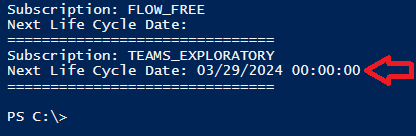
In the above image you can see Teams Exploratory license is going to expire on 29th March 2024. If the Next Life Cycle Date parameter is blank, that means the license doesn’t has an expiration date.
Export licenses expiration date to CSV file
If your requirement is to find licenses expiration date and export this information to a CSV file, run below PowerShell script:
# Get all subscriptions (licenses) in the tenant
$Subscriptions = Get-MsolSubscription
# Create an array to store the subscription information
$SubscriptionInfo = @()
# Iterate through each subscription
foreach ($Subscription in $Subscriptions) {
$SubscriptionName = $Subscription.SkuPartNumber
$NextLifeCycleDate = $Subscription.NextLifeCycleDate
# Create a custom object to store subscription details
$SubscriptionDetails = [PSCustomObject]@{
Subscription = $SubscriptionName
NextLifeCycleDate = $NextLifeCycleDate
}
# Add the custom object to the array
$SubscriptionInfo += $SubscriptionDetails
}
# Export the subscription information to a CSV file
$SubscriptionInfo | Export-Csv -Path "C:\CSV Files\SubscriptionInfo.csv" -NoTypeInformation
The above script will list the expiration date (Next Life Cycle Date) along with the license names as shown below:
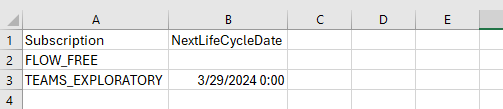
Find licenses those are going to expire in next 10 days
If you want to find the licenses those are going to expire in next 10 days, run below PowerShell script:
# Get the current date and calculate the end date (10 days from now)
$CurrentDate = Get-Date
$EndDate = $CurrentDate.AddDays(10)
# Get all subscriptions (licenses) in the tenant
$Subscriptions = Get-MsolSubscription
# Get the next life cycle date for each license
$NextLifeCycleDates = $Subscriptions | ForEach-Object {
$_.NextLifeCycleDate
}
# Check if any next life cycle date falls within the next 10 days
$ExpiringLicenses = $NextLifeCycleDates | Where-Object {
$_ -ge $CurrentDate -and $_ -le $EndDate
}
# Display expiring licenses or a message if none are found
if ($ExpiringLicenses) {
Write-Host "The following licenses are expiring within the next 10 days:"
$Subscriptions | Where-Object {
$_.NextLifeCycleDate -in $ExpiringLicenses
} | Format-Table -AutoSize
} else {
Write-Host "No licenses are expiring within the next 10 days."
}
The above script retrieves the NextLifeCycleDate for each license, checks if any of these dates fall within the next 10 days, and then displays the expiring licenses if any are found. If no licenses are expiring in the next 10 days, it displays a message “No licenses are expiring within the next 10 days”.
Find licenses those are going to expire in next 1 month
If you want to find the licenses those are going to expire in next 1 month, run below PowerShell script:
# Get the current date and calculate the end date (1 month from now)
$CurrentDate = Get-Date
$EndDate = $CurrentDate.AddMonths(1)
# Get all subscriptions (licenses) in the tenant
$Subscriptions = Get-MsolSubscription
# Get the next life cycle date for each license
$NextLifeCycleDates = $Subscriptions | ForEach-Object {
$_.NextLifeCycleDate
}
# Check if any next life cycle date falls within the next month
$ExpiringLicenses = $NextLifeCycleDates | Where-Object {
$_ -ge $CurrentDate -and $_ -le $EndDate
}
# Display expiring licenses or a message if none are found
if ($ExpiringLicenses) {
Write-Host "The following licenses are expiring within the next month:"
$Subscriptions | Where-Object {
$_.NextLifeCycleDate -in $ExpiringLicenses
} | Format-Table -AutoSize
} else {
Write-Host "No licenses are expiring within the next month."
}
The above script calculates the end date as 1 month from the current date using the AddMonths method. It then checks if any NextLifeCycleDate falls within the next month and displays the expiring licenses as shown below. If there is no license that is going to expire within a month, it will display a message “No licenses are expiring within the next month”.

Find the users who have licenses assigned (licensed users)
If you want to find the users who have licenses assigned, run below PowerShell command:
Get-MsolUser | Where-Object {$_.isLicensed -like "True"} | FT DisplayName,licenses,islicensed
The above command will list all the users who have licenses assigned along with the name of the license as shown below:
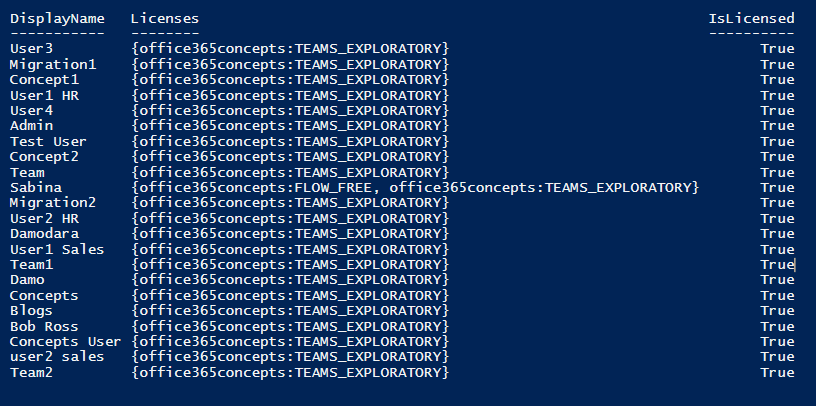
Find users who have a specific license assigned
If you want to list all the users who have a specific license assigned, use below PowerShell command:
Get-MsolUser -All | Where-Object { $_.Licenses.AccountSkuId -contains "YourLicenseSkuId" }
In the above command you need to specify the AccountSkuId of the license. To get the AccountSkuId you need to run Get-MsolAccountSku | Select-Object AccountSkuId as explained above.
Copy the AccountSkuId of the license and modify the above command. The exact command will look like below:
Get-MsolUser -All | Where-Object { $_.Licenses.AccountSkuId -contains "office365concepts:TEAMS_EXPLORATORY" }
The above PowerShell command will list all the users who have Teams Exploratory license assigned as shown below:
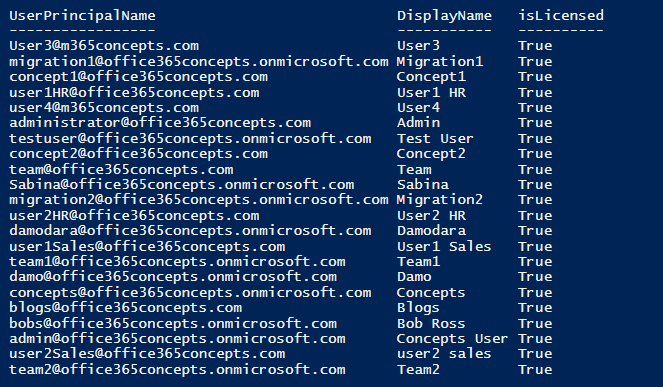
List all unlicensed users in Office 365
To find users who do not have licenses assigned, run below PowerShell command:
Get-MsolUser | Where-Object {$_.isLicensed -like "False"} | Select-Object DisplayName, isLicensed
The above command will display the users who do not have licenses assigned as shown below:
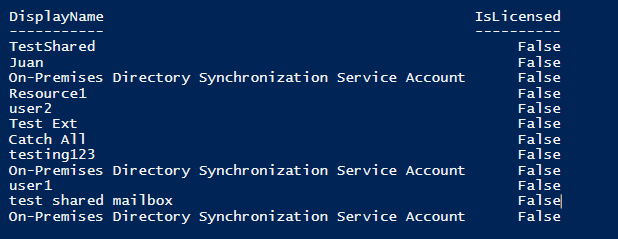
Find disabled users who have licenses assigned in Office 365 tenant
If you want to find the users whose accounts are disabled and they have licenses assigned, run below PowerShell script:
# Get all users with BlockCredential set to True and not licensed
$DisabledlicensedUsers = Get-MsolUser -All | Where-Object { $_.BlockCredential -eq $true -and $_.isLicensed -eq $True }
# Iterate through each disabled unlicensed user
foreach ($user in $DisabledlicensedUsers) {
Write-Host "User $($user.DisplayName) (UPN: $($user.UserPrincipalName)) is disabled and has license assigned."
}
The above script will display the users whose sign-in is blocked and they have licenses assigned as shown in the image below:

Conclusion
In this article you learnt how to manage Office 365 licenses with PowerShell. You might like our other articles on Manage Distribution Groups (DG) using PowerShell and Manage Dynamic Distribution Groups (DDG) using PowerShell.
If you found this article helpful and informative, please share it within your community and do not forget to share your feedback in the comments below.
Join us on YouTube for the latest videos on the Cloud technology and join our Newsletter for the early access of the updates.
Happy Scripting!!