Manage Office 365 users using Graph PowerShell
In this article you will learn how to manage Office 365 users using Graph PowerShell.
Table of Contents
Manage Office 365 users using Graph PowerShell
This guide provides a streamlined and efficient approach to administering user accounts within the Office 365 ecosystem. Leveraging the power of Microsoft Graph API and PowerShell scripting, this tool enables administrators to perform various user management tasks such as provisioning new accounts, modifying user attributes, resetting passwords, assigning licenses, and deactivating accounts, all from a command-line interface.
Create user accounts in Office 365 using PowerShell
Connect to Microsoft Graph PowerShell
Before you run below PowerShell commands, you need to connect to Microsoft Graph PowerShell. Open Windows PowerShell as Administrator and run below PowerShell commands one by one:
Set-ExecutionPolicy -ExecutionPolicy RemoteSigned -Scope CurrentUser
Install-Module Microsoft.Graph -Scope CurrentUser
Install-Module Microsoft.Graph.Beta
Connect-MgGraph -Scopes Directory.Read.All, Directory.ReadWrite.All, Organization.ReadWrite.All
Create a user using Graph PowerShell
In this example we will create a user account with name “Bob Ross” using Graph PowerShell:
$PasswordProfile = @{
Password = "Password@1"
}
New-MgUser -DisplayName "Bob Ross" -PasswordProfile $PasswordProfile -AccountEnabled -MailNickName "BobRoss" -UserPrincipalName "[email protected]"
The above command will create a new user account with DisplayName “Bob Ross” and UserPrincipalName “[email protected]”, and it will set the password for the user account. The user will be prompted to change his password on the first login attempt.
Create a user and do not force to change password using Graph PowerShell
In this example, we will create a new user account using Graph PowerShell and the user will not get a prompt to change his password.
$Password = @{
Password = "<Password>"
ForceChangePasswordNextSignIn = $false
}
New-MgUser -DisplayName "Bob Ross" -AccountEnabled -UserPrincipalName "[email protected]" -PasswordProfile $Password -MailNickname "BobRoss"
Create a user and set password never expire using Graph PowerShell
In this example we will create a new user and will set his password to never expire.
# Define user properties
$displayName = "John Smith"
$userPrincipalName = "[email protected]"
$mailNickname = "johnsmith"
$password = "P@ssw0rd123"
# Create the user
New-MgUser -DisplayName $displayName -UserPrincipalName $userPrincipalName -MailNickname $mailNickname -PasswordProfile @{"Password" = $password; "ForceChangePasswordNextSignIn" = $false} -AccountEnabled
# Get the user's ID
$userId = (Get-MgUser -Filter "userPrincipalName eq '$userPrincipalName'").Id
Bulk create users in Office 365 using Graph PowerShell
In this example, we will create multiple users using Graph PowerShell and CSV file.
Create a CSV file using below columns:
DisplayName: This column includes display name for each user.
UserPrincipalName: This column includes user name for each user.
Password: Includes password for each user account.
AccountEnabled: This column includes if account should be enabled or not.
Replace “C:\csv files\bulkusers.csv” with the actual path of your CSV file and run below Graph PowerShell script.
# Path to the CSV file
$csvFilePath = "C:\csv files\bulkusers.csv"
# Read CSV file
$users = Import-Csv -Path $csvFilePath
# Loop through each user and create them in Office 365
foreach ($user in $users) {
$mailNickname = ($user.UserPrincipalName -split '@')[0]
if ($user.AccountEnabled -eq "true") {
$accountEnabled = $true
} else {
$accountEnabled = $false
}
$newUser = @{
DisplayName = $user.DisplayName
UserPrincipalName = $user.UserPrincipalName
PasswordProfile = @{
Password = $user.Password
ForceChangePasswordNextSignIn = $false
}
AccountEnabled = $accountEnabled
MailNickname = $mailNickname
}
try {
New-MgUser @newUser -ErrorAction Stop
Write-Host "User $($user.DisplayName) created successfully." -ForegroundColor Green
} catch {
Write-Host "Failed to create user $($user.DisplayName). Error: $($_.Exception.Message)" -ForegroundColor Red
}
}
Manage user account properties using PowerShell
Disable sign in for a user using Graph PowerShell
In this example, we will disable sign in for an account in Office 365 using Graph PowerShell.
Get-MgUser -Filter "userPrincipalName eq '[email protected]'" | ForEach-Object { $_.AccountEnabled = $true; $_ | Update-MgUser -UserId $_.Id }
Bulk disable sign in for users using CSV and Graph PowerShell
In this example, we will use a CSV file to disable sign in for multiple accounts.
Create a CSV file using a column UserPrincipalName that will include the user name for each account.
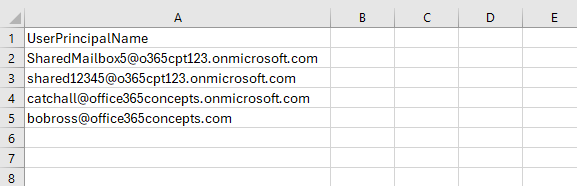
Replace “C:\Path\to\your\file.csv” with the actual path of your CSV file and run below Graph PowerShell script:
# Path to the CSV file containing user data
$csvFilePath = "C:\Path\to\your\file.csv"
# Read CSV file
$users = Import-Csv -Path $csvFilePath
# Iterate through each user in the CSV
foreach ($user in $users) {
# Get user by UserPrincipalName
$userPrincipalName = $user.UserPrincipalName
$userGraph = Get-MgUser -Filter "userPrincipalName eq '$userPrincipalName'"
# Check if user exists
if ($userGraph) {
# Disable sign-in for the user
$userGraph.AccountEnabled = $false
$userGraph | Update-MgUser -UserId $userGraph.Id
Write-Host "Disabled sign-in for user: $($userGraph.UserPrincipalName)" -ForegroundColor Green
} else {
Write-Host "User not found: $($user.UserPrincipalName)" -ForegroundColor Red
}
}
Set password never expires for a user using PowerShell
Update-MgUser –UserId "[email protected]" -PasswordPolicies DisablePasswordExpiration
Soft delete a user using Graph PowerShell
Remove-MgUser -UserId "[email protected]" -Confirm
Conclusion
In this article you learnt how to connect to Graph PowerShell, and how to manage Office 365 users using Graph PowerShell.
If you found this article helpful and informative, please share it within your community and share your feedback in the comments below.
You might like our other articles on Manage users in Office 365 using PowerShell and Manage Office 365 Licenses using PowerShell.
Join us on YouTube for the latest videos on the Cloud technology and join our Newsletter for the early access of the articles and updates.
Happy Scripting!!