Manage Room Mailbox using PowerShell
In this article you will learn how to manage Room mailbox using PowerShell commands.
Table of Contents
What is a Room Mailbox
In Microsoft Exchange Online, a room mailbox is a type of mailbox specifically designed for scheduling and managing resources such as meeting rooms or conference rooms. Room mailboxes are used to reserve these spaces for meetings, conferences, or other events. They have their own email address but are primarily utilized for managing calendar events and bookings.
Equipment mailbox vs Room mailbox
Room Mailbox: Room mailboxes are specifically designed for scheduling and managing meeting rooms or conference rooms. Room mailboxes facilitate the reservation of physical spaces for meetings, conferences, or other events. They have their own email address and calendar, allowing users to check availability and book the room for their meetings. Room mailboxes can be configured with auto-acceptance policies to automatically accept or decline meeting requests based on availability.
Equipment Mailbox: Equipment mailboxes are used for scheduling and managing resources other than meeting rooms, such as projectors, vehicles, audio-visual equipment, or any other shared equipment. Equipment mailboxes are similar to room mailboxes but are tailored for managing equipment rather than physical spaces. They also have their own email address and calendar, allowing users to schedule the use of the equipment. Like room mailboxes, equipment mailboxes can be configured with auto-acceptance policies to manage booking requests efficiently.
Manage Room Mailbox using PowerShell
Managing Room Mailbox using PowerShell allows administrators to efficiently configure and maintain room mailboxes in Microsoft Exchange Online. With PowerShell cmdlets, administrators can create new room mailboxes, modify existing ones, set booking policies, control calendar permissions, and customize settings such as capacity and location.
Connect to Exchange Online PowerShell
To be able to run below PowerShell commands, you need first connect to Exchange Online PowerShell. Run below PowerShell command in Windows PowerShell and type the credentials of a Global Administrator when prompted:
Connect-ExchangeOnline
Create Room Mailbox using PowerShell
Create a Room Mailbox using PowerShell
In this example we will create a new Room Mailbox using PowerShell. To achieve our requirement, we will use New-Mailbox PowerShell command along with Room parameter.
New-Mailbox -Room -Name "Meeting-Room1" -DisplayName "Meeting-Room1" -Alias "Meeting-Room1" -PrimarySmtpAddress "meeting-room1@office365concepts.com"
Bulk create Room Mailbox using PowerShell and CSV
In this example, we will use a CSV file to bulk create Room Mailboxes in Exchange Online.
Create a CSV file using below columns:
Name: Contains the names for each room mailbox.
DisplayName: Contains display names for each room mailbox.
PrimarySmtpAddress: Contains email address for each room mailbox.
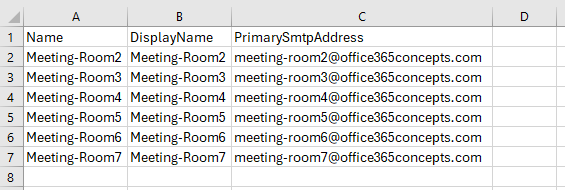
Replace “C:\csv files\bulkcreateroommailbox.csv” with the actual path of your CSV file, and run below PowerShell script:
# Path to the CSV file
$csvFilePath = "C:\csv files\bulkcreateroommailbox.csv"
# Import the data from CSV
$mailboxData = Import-Csv $csvFilePath
# Iterate through each row in the CSV and create room mailboxes
foreach ($row in $mailboxData) {
$name = $row.Name
$displayName = $row.DisplayName
$primarySMTPAddress = $row.PrimarySMTPAddress
# Create the room mailbox
New-Mailbox -Room -Name $name -DisplayName $displayName -PrimarySmtpAddress $primarySMTPAddress
Write-Host "Room mailbox '$displayName' created."
}
The above PowerShell script will iterate through each row in the CSV file, extracting the values for Name, DisplayName, and PrimarySMTPAddress, and then use those values to create room mailboxes using the New-Mailbox cmdlet along with Room parameter.
Manage Room Mailbox properties using PowerShell
Enable Room Mailbox auto accept meeting requests
If a room mailbox doesn’t auto accept meetings, you need to update the Room Mailbox’ calendar properties to AutoAccept. To achieve this, we will use Set-CalendarProcessing PowerShell command along with AutomateProcessing parameter.
Set-CalendarProcessing -Identity "Meeting-Room1" -AutomateProcessing AutoAccept
To verify if room mailbox calendar’s AutomateProcessing is set to AutoAccept, run below PowerShell command:
Get-CalendarProcessing -Identity "Meeting-Room1" | Select-Object AutomateProcessing
Bulk enable Room Mailbox auto accept meeting requests
If you want to enable all the room mailboxes to automatically accept the meeting invitations, run below PowerShell command:
Get-Mailbox | Where-Object {$_.RecipientTypeDetails -eq "RoomMailbox"} | Set-CalendarProcessing -AutomateProcessing AutoAccept
Enable Room Mailbox to auto accept external meetings / internal meeting received through inbound connector
You can come across scenarios, where you schedule a meeting with users who are internal to your organization, and you specify a meeting room in the meeting invitation, the room mailbox for the meeting room doesn’t accept or decline the meeting automatically even if it’s set up to automatically accept the meetings.
This happens because the room mailbox treats the invitation that’s received from the third-party mail service through the inbound connector as an external message. To fix this, you need to enable ProcessExternalMeetingMessages parameter of the room mailbox.
Set-CalendarProcessing -Identity "Meeting-Room1" -ProcessExternalMeetingMessages $true
Bulk enable Room Mailbox to auto accept external meetings / internal meeting received through inbound connector
To enable ProcessExternalMeetingMessages parameter for all the Room Mailboxes in Exchange Online, run below PowerShell command:
Get-Mailbox | Where-Object {$_.RecipientTypeDetails -eq "RoomMailbox"} | Set-CalendarProcessing -ProcessExternalMeetingMessages $true
The above PowerShell command will enable all the room mailboxes to auto accept external meetings or the internal meetings those are received over inbound connector.
Set Room Mailbox to allow conflicts
To enable a room mailbox to allow conflicting meetings, run below PowerShell command:
Set-CalendarProcessing -Identity "Meeting-Room1" -AllowConflicts $true
Bulk allow conflicts on all Room Mailboxes
To enable all room mailboxes to allow conflicting meetings, run below PowerShell command:
Get-Mailbox | Where-Object {$_.RecipientTypeDetails -eq "RoomMailbox"} | Set-CalendarProcessing -AllowConflicts $true
Set Room Mailbox capacity using PowerShell
A room mailbox’s capacity defines the maximum number of people who can safely occupy the room. To set room mailbox capacity, run below PowerShell command:
Set-Mailbox -Identity "Meeting-Room1" -ResourceCapacity 20
To verify capacity of a room mailbox, run below PowerShell command:
Get-Mailbox -Identity "Meeting-Room1" | Select-Object ResourceCapacity
Hide Room Mailbox in Global Address List
To hide a room mailbox in Global Address List (GAL), run below PowerShell command:
Set-Mailbox -Identity "Meeting-Room1" -HiddenFromAddressListsEnabled $true
Set working hours for Room Mailbox / Enable room mailbox to accept meetings scheduled in working hours
We can enable a room mailbox to accept or decline the meeting requests that aren’t booked during the working hours defined for the room. By default, this setting is disabled, so meeting requests are allowed outside the working hours. By default, working hours are 8:00 A.M. to 5:00 P.M. Monday through Friday.
To enable a room mailbox to accept meetings only within working hours, run below PowerShell command:
Set-CalendarProcessing -Identity "Meeting-Room1" -ScheduleOnlyDuringWorkHours $true
Set working hours for all Room Mailboxes
To enable all the room mailboxes to accept meetings only in working hours, run below PowerShell command:
Get-Mailbox | Where-Object {$_.RecipientTypeDetails -eq "RoomMailbox"} | Set-CalendarProcessing -ScheduleOnlyDuringWorkHours $true
Assign delegate to Room Mailbox
To assign a delegate to the room mailbox that will be responsible to approve all the meetings of a room mailbox, run below PowerShell command:
Set-CalendarProcessing -Identity "Meeting-Room1" -ResourceDelegates "Concept1","concept2"
The above PowerShell command will set users “Concept1” and “Concept2” as delegates for room mailbox “Meeting-Room1”.
Allow Room Mailbox to forward meeting requests to delegates
In this example we will allow room mailbox to forward all incoming meeting invites to delegates.
Set-CalendarProcessing -Identity "Meeting-Room1" -ForwardRequestsToDelegates $true
How to fix when Room Mailbox meetings show organizer name instead of subject of the meeting
Set-CalendarProcessing -Identity "Meeting-Room1" -AddOrganizerToSubject $False -DeleteSubject $False
Create a Room List
A Room List is used to organize the Room Mailboxes. If your company has several buildings with rooms that can be booked for meetings, you can create room lists for each building to manage the room mailboxes. Room lists are the distribution groups and can be managed only using PowerShell.
To create a Room List, run below PowerShell command:
New-DistributionGroup -Name "Building-1 Rooms" -RoomList
Next, we will add Room Mailboxes within the above Room List:
Add-DistributionGroupMember -Identity "Building-1 Rooms" -Member "Meeting-Room1"
Important: The maximum number of rooms that can be returned in a request for a Room List is 100. This also includes when you are using Microsoft Teams to create a meeting invite. A possible workaround would be to further break down your rooms into smaller lists.
Convert Room Mailbox
Convert Room Mailbox to Shared Mailbox
To convert a Room Mailbox to Shared Mailbox, run below PowerShell command:
Set-Mailbox -Identity "Meeting-Room1" -Type Shared
Convert Room Mailbox to User Mailbox (Regular Mailbox)
To convert a Room Mailbox to User Mailbox, run below PowerShell command:
Set-Mailbox -Identity "Meeting-Room2" -Type Regular
Conclusion
In this article you learnt how to manage Room Mailbox using PowerShell commands. You learnt how to manage Room Mailbox auto accept meeting requests, how to set Room Mailbox capacity using PowerShell, and how to create a Room List using PowerShell commands.
If you found this article helpful and informative, please share it within your community and share your feedback in the comments below.
You might like our other articles on Manage Users in Office 365 using PowerShell, Manage Shared Mailbox using PowerShell and Manage Office 365 licenses using PowerShell.
Please join us on YouTube for the latest videos on the Cloud technology and join our Newsletter for the early access of the articles and updates.
Happy Scripting!!