Office 365 license management with Graph PowerShell
This article is dedicated to Office 365 license management with Graph PowerShell. In this article you will learn how to assign Office 365 licenses using Graph PowerShell, how to assign and remove licenses using Graph PowerShell, and how to create license reports using Graph PowerShell.
Table of Contents
Assign Office 365 licenses with Graph PowerShell
Connect to Microsoft Graph PowerShell
To be able to run below Graph PowerShell commands, you need to connect to Microsoft Graph PowerShell module. Open Windows PowerShell as administrator and run below commands one by one:
Set-ExecutionPolicy -ExecutionPolicy RemoteSigned -Scope CurrentUser
Install-Module Microsoft.Graph -Scope CurrentUser
Install-Module Microsoft.Graph.Beta
Connect-MgGraph -Scopes Directory.Read.All, Directory.ReadWrite.All, Organization.ReadWrite.All
List all Office 365 licenses using Graph PowerShell
To find all licenses in Office 365 tenant using Graph PowerShell, run below command:
Get-MgSubscribedSku | Select-Object SkuId,SkuPartNumber,ServicePlans,ConsumedUnits
The above command will list all licenses in Office 365 tenant as shown below:

If you want to list all licenses along with all their properties, run below Graph PowerShell command:
Get-MgSubscribedSku | FL
Check status of all licenses using Graph PowerShell
To check status of all licenses in Office 365 tenant, run below command:
# Get all subscribed SKUs (license plans)
$licenses = Get-MgSubscribedSku
# Loop through each license plan and display details
foreach ($license in $licenses) {
Write-Host "License SKU: $($license.SkuId)"
Write-Host " - Enabled: $($license.Enabled)"
Write-Host ""
}
Assign Office 365 license using Graph PowerShell
In this example we will assign license to a user using Graph PowerShell.
To assign license using Graph PowerShell, we need SkuId of the license that we want to assign to the user. To get the SkuId of the subscriptions, run below command:
Get-MgSubscribedSku | Select-Object SkuId,SkuPartNumber
The above command will list all subscriptions along with their SkuId and SkuPartNumber as shown below:

If you want to find SkuId for a particular license, run below Graph PowerShell command:
Get-MgSubscribedSku -All | Where SkuPartNumber -eq "O365_BUSINESS_ESSENTIALS" | Select-Object SkuId
The above command will list SkuId of O365_BUSINESS_ESSENTIALS (Microsoft 365 Business Basic) license.
Important: When you manage licenses using PowerShell, you find the license names less friendly. You can find all the license names in this article.
Once you have required SkuId of the license, run below command to assign license to the user.
Set-MgUserLicense –UserId "[email protected]" -AddLicenses @{SkuId = "3b555118-da6a-4418-894f-7df1e2096870"} -RemoveLicenses @()
Important: You can not assign license to an account whose Usage Location is not updated. If you try to assign license to an account without updating usage location, you will get error that says “Set-MgUserLicense : License assignment cannot be done for user with invalid usage location.“
To update Usage Location for an account, run below Graph PowerShell command:
Update-MgUser -UserId "[email protected]" -UsageLocation IN
Assign multiple licenses to a user using Graph PowerShell
In this example we will assign multiple licenses to an account using graph PowerShell.
Type UPN of the account to whom you want to assign licenses, type the SkuId of the licenses as shown below, and run below Graph PowerShell script.
# Define user UPN and license SkuIDs (replace with actual values)
$userUpn = "[email protected]"
$licenseSkuId1 = "f30db892-07e9-47e9-837c-80727f46fd3d"
$licenseSkuId2 = "3b555118-da6a-4418-894f-7df1e2096870" # Add more as needed
# Create hash tables for each license
$license1 = @{ SkuId = $licenseSkuId1 }
$license2 = @{ SkuId = $licenseSkuId2 }
# Combine licenses into an array
$licenses = @($license1, $license2) # Add more license hash tables to the array
# Assign licenses and remove any existing ones
Set-MgUserLicense -UserId $userUpn -AddLicenses $licenses -RemoveLicenses @()
Assign license to multiple users using CSV and Graph PowerShell
In this example we will use a CSV file and Graph PowerShell to assign license to multiple users.
Create a CSV file with below columns:
UserId: This column will include the User Principal Name of each user to whom you want to assign license.
SkuId: This column will include SkuId of each license you want to assign to the users.
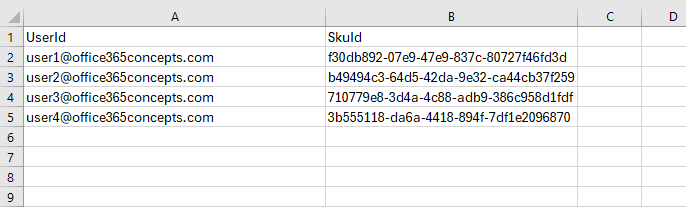
Replace “C:\CSV Files\bulklicense.csv” with the actual path of the CSV file and run below Graph PowerShell script:
# Path to your CSV file (replace with the actual path)
$csvFilePath = "C:\CSV Files\bulklicense.csv"
# Import CSV data
$userLicenses = Import-Csv -Path $csvFilePath
# Loop through each user-license entry
foreach ($entry in $userLicenses) {
# Extract user ID and license SkuId
$userId = $entry.UserId
$licenseSkuId = $entry.SkuId
# Create a hash table for the license
$license = @{ SkuId = $licenseSkuId }
# Assign the license to the user
try {
Set-MgUserLicense -UserId $userId -AddLicenses $license -RemoveLicenses @()
Write-Host "Successfully assigned license '$licenseSkuId' to user '$userId'."
} catch {
Write-Warning "Failed to assign license to user '$userId': $($_.Exception.Message)"
}
}
Assign multiple licenses to multiple users using CSV and Graph PowerShell
In this example we will assign multiple licenses to multiple users using CSV file and Graph PowerShell script.
Create a CSV file with below columns:
UserId: This column will include the User Principal Name of each user to whom you want to assign license.
SkuId1: This column will include SkuId of license you want to assign to the users.
SkuId2: This column will include SkuId of another license you want to assign to the user.
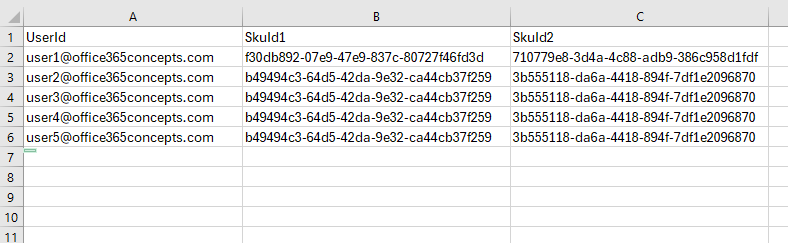
Replace “C:\CSV Files\bulklicenses.csv” with the actual path of your CSV file and run below Graph PowerShell script:
# Path to your CSV file (replace with the actual path)
$csvFilePath = "C:\CSV Files\bulklicense.csv"
# Import CSV data
$userLicenses = Import-Csv -Path $csvFilePath
# Loop through each user-license entry
foreach ($entry in $userLicenses) {
# Extract user ID and license SkuIds
$userId = $entry.UserId
$licenseSkuId1 = $entry.SkuId1
$licenseSkuId2 = $entry.SkuId2 # Add more license properties as needed (e.g., SkuId3)
# Create an array to hold license hash tables
$licenses = @()
# Check for presence of SkuId1 and add license hash table if exists
if ($licenseSkuId1) {
$license1 = @{ SkuId = $licenseSkuId1 }
$licenses += $license1
}
# Check for presence of SkuId2 and add license hash table if exists
if ($licenseSkuId2) {
$license2 = @{ SkuId = $licenseSkuId2 }
$licenses += $license2
}
# Assign licenses (if any) to the user
if ($licenses.Count -gt 0) {
try {
Set-MgUserLicense -UserId $userId -AddLicenses $licenses -RemoveLicenses @()
Write-Host "Successfully assigned licenses to user '$userId'."
} catch {
Write-Warning "Failed to assign licenses to user '$userId': $($_.Exception.Message)"
}
} else {
Write-Warning "No licenses found for user '$userId'."
}
}
Assign same license from one user to another using Graph PowerShell
In this example we will assign same license to a user that is assigned to another user. We have a user [email protected] that has Microsoft Power Automate Free and Microsoft Teams Exploratory licenses assigned. We will use Graph PowerShell to assign same licenses to [email protected].
In this scenario, source user will be “Bob Ross”, and target user will be “John”. Replace source user ($sourceUserId) and target user ($targetUserId) and run below Graph PowerShell script:
# Define UserIDs (replace with actual values)
$sourceUserId = "[email protected]" # User with the license to copy
$targetUserId = "[email protected]" # User to assign the license to
# Get assigned licenses for the source user
$sourceLicenses = Get-MgUserLicenseDetail -UserId $sourceUserId | Select-Object SkuId
# Check if licenses were found
if ($sourceLicenses) {
# Loop through each license SkuId
foreach ($licenseSkuId in $sourceLicenses.SkuId) {
# Assign the license to the target user
try {
Set-MgUserLicense -UserId $targetUserId -AddLicenses @{ SkuId = $licenseSkuId } -RemoveLicenses @()
Write-Host "Successfully assigned license '$licenseSkuId' to user '$targetUserId'."
} catch {
Write-Warning "Failed to assign license to user '$targetUserId': $($_.Exception.Message)"
}
}
} else {
Write-Warning "No licenses found for source user '$sourceUserId'."
}
Assign license to each member of a group using Graph PowerShell
In this example we will use Graph PowerShell to assign license to each member of a security group.
First, we will collect Group Id of the security group by running Get-MgGroup command.
Get-MgGroup | Select-Object DisplayName,Id
The above command will list all the groups in your Office 365 tenant along with their display name and group Ids as shown below:
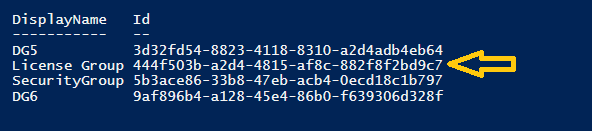
In this scenario we will use the Group Id of “License Group” to assign license to each member of the group.
Modify GroupId and SkuId and run below Graph PowerShell command:
Set-MgGroupLicense -GroupId "444f503b-a2d4-4815-af8c-882f8f2bd9c7" -AddLicenses @{SkuId = "3b555118-da6a-4418-894f-7df1e2096870"} -RemoveLicenses @()
Conclusion
In this article you learnt how to assign Office 365 licenses with Graph PowerShell, you learnt how to assign multiple licenses to multiple users using CSV file, assign multiple licenses to a user, assign same license from one user to another, and how to assign license to each member of a group using Graph PowerShell.
If you found this article helpful and informative, please share it within your community and do not forget to share your feedback in the comments below.
You might like our other articles on How to connect to Graph PowerShell and Manage Office 365 users using Graph PowerShell.
Join us on YouTube for the latest videos on the Cloud technology and join our Newsletter for the early access of the articles and updates.
Happy Scripting!!